基于华为鲲鹏云服务器CentOS中(或Ubuntu),使用Linux Socket实现:
1. Web服务器的客户端服务器,提交程序运行截图
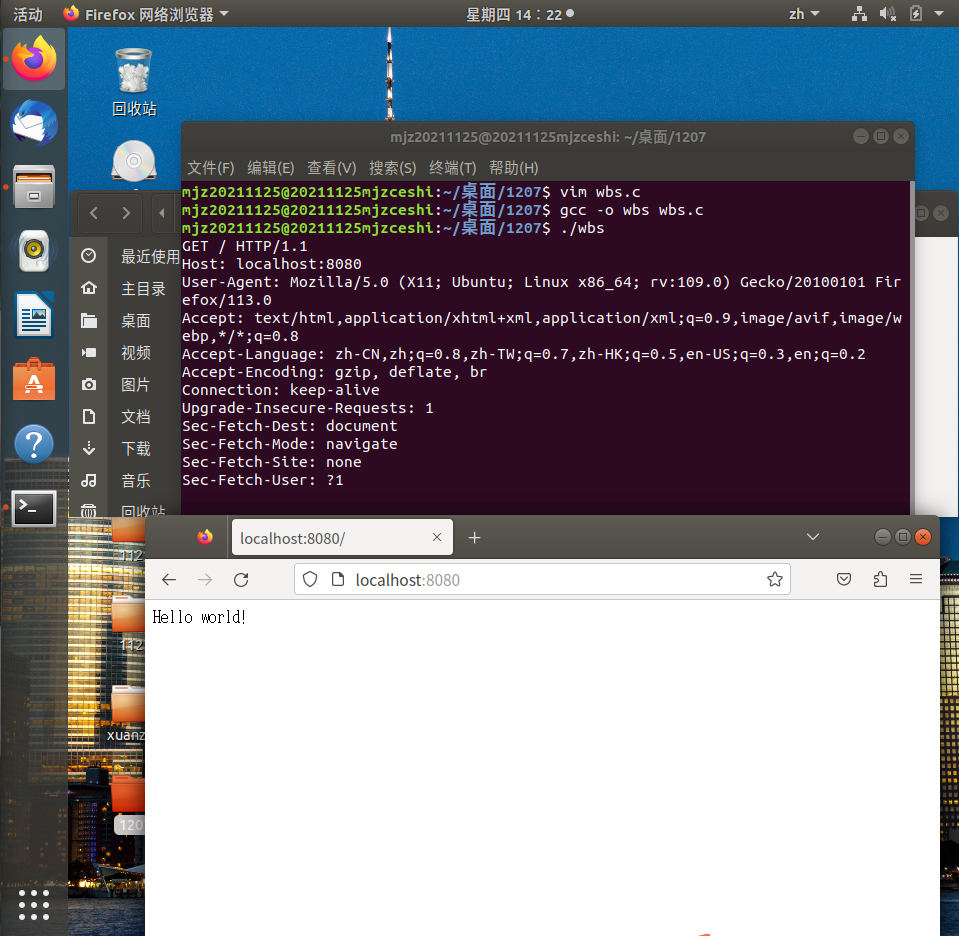
2. 实现GET即可,请求,响应要符合HTTP协议规范
3. 服务器部署到华为云服务器,浏览器用本机的
4. 把服务器部署到试验箱。(加分项)
Web服务器代码(wbs.c)
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/socket.h>
#include <netinet/in.h>
#define PORT 8080
int main() {
int server_fd, new_socket;
struct sockaddr_in address;
int addrlen = sizeof(address);
char buffer[30000] = {0};
char *hello = "HTTP/1.1 200 OK\nContent-Type: text/html\nContent-Length: 13\n\nHello world!";
// Creating socket file descriptor
server_fd = socket(AF_INET, SOCK_STREAM, 0);
if (server_fd == 0) {
perror("socket failed");
exit(EXIT_FAILURE);
}
// Setting up the address structure
address.sin_family = AF_INET;
address.sin_addr.s_addr = INADDR_ANY;
address.sin_port = htons(PORT);
// Bind the socket to the address
if (bind(server_fd, (struct sockaddr *)&address, sizeof(address)) < 0) {
perror("bind failed");
exit(EXIT_FAILURE);
}
// Listen for connections
if (listen(server_fd, 3) < 0) {
perror("listen");
exit(EXIT_FAILURE);
}
// Accept a connection
if ((new_socket = accept(server_fd, (struct sockaddr *)&address, (socklen_t*)&addrlen)) < 0) {
perror("accept");
exit(EXIT_FAILURE);
}
// Read the incoming message
read(new_socket, buffer, 30000);
printf("%s\n", buffer);
// Send the response
write(new_socket, hello, strlen(hello));
close(new_socket);
return 0;
}