右侧搜索根据条件查找到对应人,人再查询到对应部门。
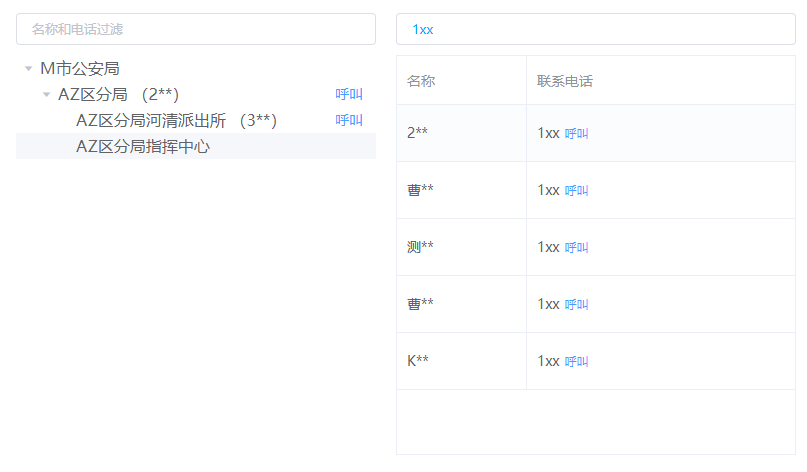
<template>
<div class="contact_tree">
<el-input v-model="filterText" size="small" placeholder="名称和电话过滤" clearable />
<el-tree class="contact_tree1" :data="tree" node-key="id" ref="tree" default-expand-all
:filter-node-method="filterNode" :expand-on-click-node="false" @node-click="nodeClick">
<template #default="{ data }">
<span class="custom-tree-node">
<p class="tree_name" :title="data.lxdh ? `联系电话:${data.lxdh}` : ''">{{ data.value }} {{ data.lxdh ?
`(${data.lxdh})` : "" }}</p>
<el-button style="position: absolute;right: 12px;" v-if="data.lxdh" type="text"
@click.stop="callDH(data)">呼叫</el-button>
</span>
</template>
</el-tree>
</div>
<div class="contact_list">
<el-input v-model="filterPolice" placeholder="名称和电话过滤" clearable size="small" />
<div style="margin-top: 10px;">
<el-table :data="list" height="400" border style="width: 100%">
<el-table-column prop="yhmc" label="名称" width="130"> </el-table-column>
<el-table-column prop="lxdh" label="联系电话">
<template #default="scope">
<div v-if="scope.row.lxdh">
{{ scope.row.lxdh }}
<el-button v-show="scope.row.lxdh" link type="text" size="small"
@click="callDH(scope.row)">呼叫</el-button>
</div>
<div v-else>暂无号码</div>
</template>
</el-table-column>
</el-table>
</div>
</div>
</template>
<script>
import { mapGetters, mapState } from "vuex";
import { setStore, getStore, clearStore } from "@/util/store";
import { read, utils } from "xlsx";
export default {
data() {
return {
tree: [],
outTree: [],
list: [],
outList: [],
police: {},
filterText: "",
filterPolice: "",
currNode: "",
currNode2: "",
activeTab: "1",
addVis: false,
importExcel: [],
uploadShow: true,
formFZ: {
fid: "",
mc: "",
},
formDW: {
ssfzid: "",
dwmc: "",
lxdh: "",
},
formRY: {
fid: "",
lxrmc: "",
lxdh: "",
lxdz: "",
},
rules: {
mc: [{ required: true, message: "请输入分组名称", trigger: "blur" }],
dwmc: [{ required: true, message: "请输入单位名称", trigger: "blur" }],
lxrmc: [{ required: true, message: "请输入联系人名称", trigger: "blur" }],
lxdh: [{ required: true, message: "请输入联系电话", trigger: "blur" }],
},
};
},
mounted() {
this.$store.dispatch("contacts/getDept").then((v) => {
this.tree = v;
});
let police = getStore({ name: "policeman" });
police.forEach((v) => {
this.police[v.dwbh] ? "" : (this.police[v.dwbh] = []);
if (v.yhmc) this.police[v.dwbh].push(v);
});
this.getFZList();
},
watch: {
filterText(val) {
this.$refs.tree.filter(val);
},
filterPolice(val) {
this.filterDeptByKeywold(val);
},
},
methods: {
callDH(row) {
if (row && row.lxdh) this.$emitter.emit("changeDHHM", row.lxdh);
else this.$message.warning("暂无电话");
},
closeBox() {
this.$store.commit("contacts/SET_CONTACTSVIS", false);
},
getList(val) {
if (val) {
let arr = this.police[this.currNode] || [];
this.list = arr.filter((v) => {
return (v.yhmc && v.yhmc.indexOf(val) !== -1) || (v.lxdh && v.lxdh.indexOf(val) !== -1);
});
} else {
this.list = this.police[this.currNode] || [];
}
},
filterNode(value, data) {
if (!value) return true;
// 根据右边的数组对象结果筛选左边的部门
if (Object.prototype.toString.call(value) === "[object Array]") {
let target = value.find((s) => s.dwbh === data.key);
if (target) {
return true;
}
}
let is = data.value.indexOf(value) !== -1 || (data.lxdh && data.lxdh.indexOf(value) !== -1);
return is;
},
// 根据关键字查找右侧联系人并找到对应的部门
filterDeptByKeywold(val) {
if (val) {
// 根据keyword查找指定对象
let temp = [];
for (const key in this.police) {
if (Object.hasOwnProperty.call(this.police, key)) {
const item = this.police[key];
let target = item.filter((s) => (s.lxdh && s.lxdh.includes(val)) || (s.yhmc && s.yhmc.includes(val)));
if (target) {
temp = temp.concat(target);
}
}
}
this.list = temp;
// 查找到则去找到对应部门,未查找到则重置左侧部门数据
if (this.list.length === 0) {
this.$refs.tree.filter("");
} else {
this.$refs.tree.filter(this.list);
}
console.log(this.list, "this.list");
} else {
this.$refs.tree.filter(val);
this.list = [];
}
},
nodeClick(data) {
this.currNode = data.key;
this.getList();
},
// 外部过滤
filterNode2(value, data) {
if (!value) return true;
let is = (data.mc && data.mc.indexOf(value) !== -1) || (data.dwmc && data.dwmc.indexOf(value) !== -1) || (data.lxdh && data.lxdh.indexOf(value) !== -1);
return is;
},
nodeClick2(data) {
if (data && data.lx == "0") {
this.currNode2 = data;
this.filterPolice2 = "";
this.getRYList();
} else {
this.$store.commit("contacts/SET_WBLISTRY", []);
}
},
// 获取左侧分组
getFZList() {
this.$store.dispatch("contacts/getTXLDW").then((v) => { });
},
// 获取右侧联系人
getRYList() {
this.$store
.dispatch("contacts/getTXLRY", {
dwid: this.currNode2.id,
keyWord: this.filterPolice2,
})
.then((v) => { });
},
},
};