[LeetCode Hot 100] LeetCode148. 排序链表
发布时间 2023-12-11 21:06:27作者: Ac_c0mpany丶
题目描述
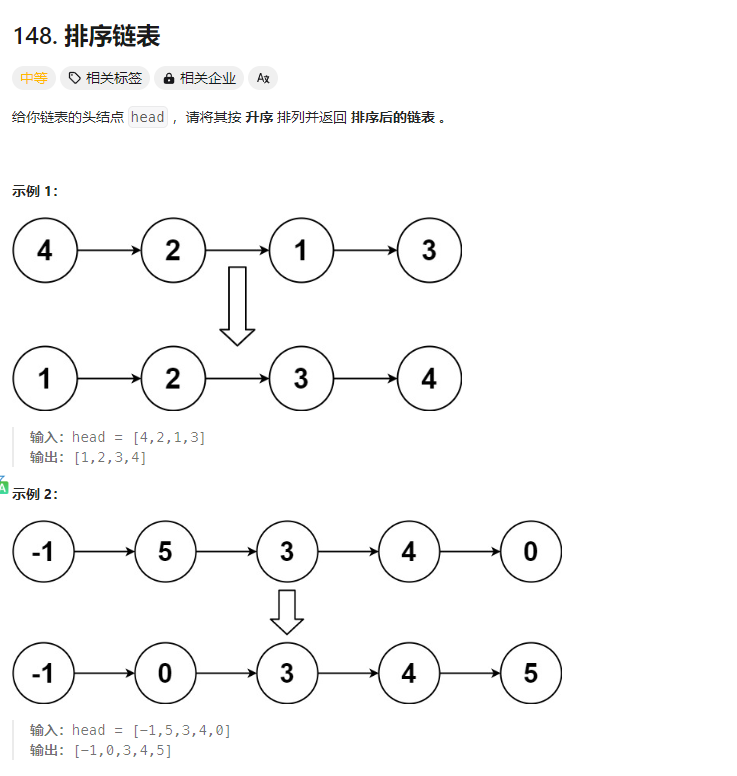
思路一:堆排序、小顶堆
- 定义一个最小堆
- 将链表的所有节点放入一个最小堆中
- 直接用队列弹出的最小值依次覆盖掉原链表的值
方法一:
/**
* Definition for singly-linked list.
* public class ListNode {
* int val;
* ListNode next;
* ListNode() {}
* ListNode(int val) { this.val = val; }
* ListNode(int val, ListNode next) { this.val = val; this.next = next; }
* }
*/
class Solution {
public ListNode sortList(ListNode head) {
// 定义一个最小堆
PriorityQueue<Integer> heap = new PriorityQueue<>();
ListNode cur = head;
ListNode dummy = new ListNode();
// 将链表的所有节点放入一个最小堆中
while (cur != null) {
heap.offer(cur.val);
cur = cur.next;
}
cur = head;
// 直接用队列弹出的最小值依次覆盖掉原链表的值
while (!heap.isEmpty()) {
cur.val = heap.poll();
cur = cur.next;
}
return head;
}
}