顺序栈的基本模型
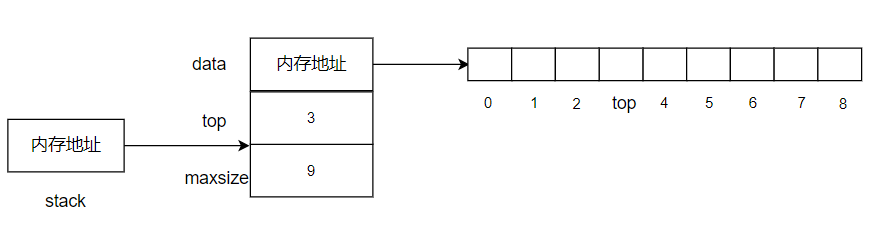
完整的C代码
点击查看代码
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
typedef struct StackNode {
int* data;
int top;
int maxsize;
} StackNode, *Stack;
Stack createStack(int maxsize);
bool isEmpty(Stack stack);
bool isFull(Stack stack);
bool push(Stack stack, int element);
int pop(Stack stack);
void destroyStack(Stack stack);
int main(int argc, char* argv[]) {
Stack stack = createStack(10);
push(stack, 32);
push(stack, 16);
push(stack, 20);
push(stack, 18);
printf("top = %d\n", stack->top);
printf("popped value = %d\n", pop(stack));
printf("top = %d\n", stack->top);
return 0;
}
Stack createStack(int maxsize) {
Stack stack = (Stack)malloc(sizeof(StackNode));
stack->data = (int*)malloc(sizeof(int) * maxsize);
stack->top = -1;
stack->maxsize = maxsize;
return stack;
}
bool isEmpty(Stack stack) {
return stack->top == -1;
}
bool isFull(Stack stack) {
return stack->top == stack->maxsize - 1;
}
bool push(Stack stack, int element) {
if (isFull(stack)) {
printf("Ths stack is full!\n");
return false;
} else {
(stack->data)[++stack->top] = element;
return true;
}
}
int pop(Stack stack) {
if (isEmpty(stack)) {
printf("Ths stack is empty!");
return 99999;
} else {
return (stack->data)[stack->top--];
}
}
void destroyStack(Stack stack) {
free(stack->data);
stack->data = NULL;
free(stack);
stack = NULL;
}