TextCoder.hpp

1 #include <string> 2 3 class TextCoder { 4 private: 5 std::string text; 6 7 void encoder() { 8 for (char& c : text) { 9 if (c >= 'a' && c <= 'z') { 10 c = ((c - 'a' + 7) % 26) + 'a'; 11 } else if (c >= 'A' && c <= 'Z') { 12 c = ((c - 'A' + 7) % 26) + 'A'; 13 } 14 } 15 } 16 17 void decoder() { 18 for (char& c : text) { 19 if (c >= 'a' && c <= 'z') { 20 c = ((c - 'a' + 19) % 26) + 'a'; 21 } else if (c >= 'A' && c <= 'Z') { 22 c = ((c - 'A' + 19) % 26) + 'A'; 23 } 24 } 25 } 26 27 public: 28 TextCoder() = default; 29 TextCoder(const std::string& t) : text(t) {} 30 31 std::string get_ciphertext() { 32 encoder(); 33 return text; 34 } 35 36 std::string get_deciphertext() { 37 decoder(); 38 return text; 39 } 40 };
task5.cpp

1 #include "textcoder.hpp" 2 #include <iostream> 3 #include <string> 4 5 void test() { 6 using namespace std; 7 8 string text, encoded_text, decoded_text; 9 10 cout << "输入英文文本: "; 11 while (getline(cin, text)) { 12 encoded_text = TextCoder(text).get_ciphertext(); 13 cout << "加密后英文文本:\t" << encoded_text << endl; 14 15 decoded_text = TextCoder(encoded_text).get_deciphertext(); 16 cout << "解密后英文文本:\t" << decoded_text << endl; 17 cout << "\n输入英文文本: "; 18 } 19 } 20 21 int main() { 22 test(); 23 }
运行结果截图
Info.hpp

1 #pragma once 2 3 #include<iostream> 4 #include<string> 5 #include <iomanip> 6 7 using namespace std; 8 class Info{ 9 public: 10 Info(string nickname0, string contact0, string city0, int n0); 11 ~Info()=default; 12 13 void print() const; 14 15 private: 16 string nickname,contact,city; 17 int n; 18 }; 19 20 Info::Info(string nickname0, string contact0, string city0, int n0) 21 { 22 nickname = nickname0; 23 contact = contact0; 24 city = city0; 25 n=n0; 26 } 27 28 void Info::print() const 29 { 30 cout << "昵称: " << nickname << endl; 31 cout << "联系方式: " << contact << endl; 32 cout << "所在城市: " << city << endl; 33 cout << "预定参加人数: " << n << endl; 34 }
task6.cpp

1 #include<iostream> 2 #include<string> 3 #include<vector> 4 #include "info.hpp" 5 6 const int capacity = 100; 7 8 9 int main() 10 { 11 vector<Info> audience_info_list; 12 13 cout << "录入信息:" << endl; 14 cout << endl; 15 cout << "昵称 联系方式(邮箱/手机号) 所在城市 预定参加人数 " << endl; 16 char choice; 17 int sum=0; 18 string name, contact, city; 19 int n; 20 while(cin >> name >> contact >> city >> n) 21 { 22 sum+=n; 23 24 if(sum > capacity) 25 { 26 sum-=n; 27 cout << "对不起,只剩" << capacity-sum << "个位置" << endl; 28 cout << "1.输入u,更新(update)预定信息" << endl 29 << "2.输入q, 退出预定" << endl 30 <<"你的选择: "; 31 cin >> choice; 32 33 if(choice=='u') 34 { 35 cin >> name >> contact >> city >> n; 36 Info a(name,contact,city,n); 37 audience_info_list.push_back(a); 38 sum+=n; 39 } 40 else 41 break; 42 } 43 else audience_info_list.push_back(Info(name, contact, city, n)); 44 } 45 cout << "截至目前,一共有" << sum << "位听众预定参加。预定听众信息如下:" << endl; 46 for(auto &Info:audience_info_list) 47 { 48 Info.print(); 49 } 50 }
运行结果截图
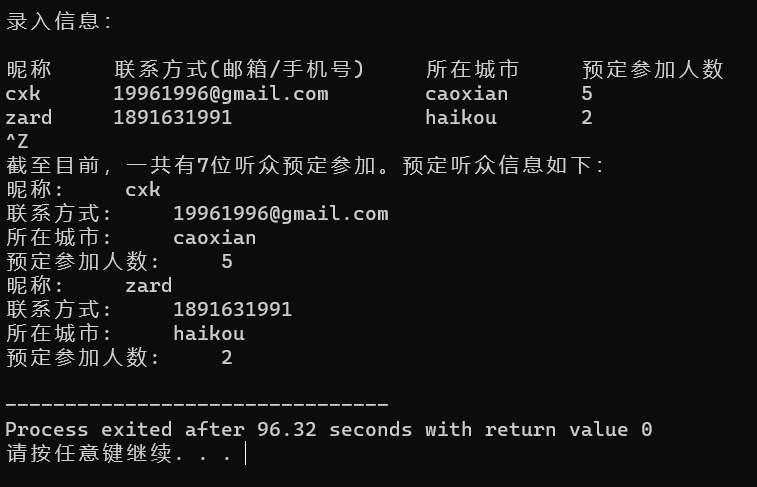