实验任务5
task5.cpp
#include"Textcoder.hpp"
#include<iostream>
#include<string>
void test()
{
using namespace std;
string text, encoded_text, decoded_text;
cout << "输入英文文本: ";
while (getline(cin, text))
{
encoded_text= TextCoder(text).get_ciphertext();
cout << "加密后英文文本:\t" << encoded_text << endl;
decoded_text = TextCoder(encoded_text).get_deciphertxet();
cout << "解密后英文文本:\t" << decoded_text << endl;
cout << "\n输入英文文本: ";
}
}
int main()
{
test();
}
TextCoder.cpp
#include"TextCoder.hpp"
#include <ctype.h>
using namespace std;
TextCoder::TextCoder(const std::string& input_text) : text(input_text){}
void TextCoder::encoder()
{
for (char& c : text)
{
if (isalpha(c))
{
if (islower(c))
{
c = 'a'+(c - 'a' + 7) % 26;
}
else {
c = 'A'+(c - 'A' + 7) % 26;
}
}
}
}
void TextCoder::decoder() {
for (char& c : text) {
if (isalpha(c))
{
if (islower(c)) {
c = 'a' + (c - 'a' - 7 + 26) % 26;
}
else {
c = 'A' + (c - 'A' - 7 + 26) % 26 ;
}
}
}
}
string TextCoder::get_ciphertext() {
encoder();
return text;
}
string TextCoder::get_deciphertxet(){
decoder();
return text;
}
TextCoder.hpp
#ifndef TEXTCODER_HPP
#define TEXTCODER_HPP
#include <string>
using namespace std;
class TextCoder
{
private:
string text;
void encoder();
void decoder();
public:
TextCoder(const string& input_text);
string get_ciphertext();
string get_deciphertxet();
};
#endif
运行结果
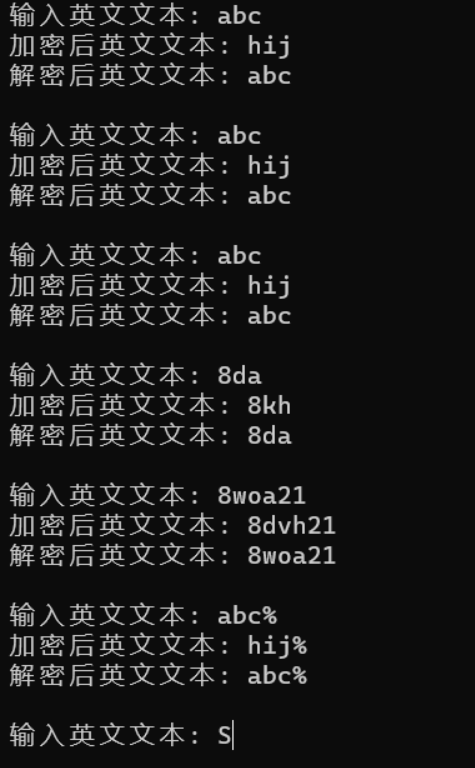
实验任务6
info.hpp
#pragma once
#include <iostream>
#include <string>
using namespace std;
class Info {
private:
string nickname;
string contact;
string city;
int n;
public:
Info(const string& nickname, const string& contact, const string& city, const int& n) : nickname{ nickname }, contact{ contact }, city{ city }, n{ n }
{};
void print() const
{
cout << "昵称:\t\t" << nickname << endl;
cout << "联系方式:\t" << contact << endl;
cout << "所在城市:\t" << city << endl;
cout << "预定人数:\t" << n << endl;
}
};
task6.cpp
#include <vector>
#include "info.hpp"
using namespace std;
int main() {
const int capacity = 100;
int current_number = 0;
string nickname, contact, city;
int n;
vector<Info> audience_info_list;
vector<Info>& v = audience_info_list;
cout << "录入信息:\n";
cout << "昵称\t\t";
cout << "联系方式(邮箱/手机号)\t\t";
cout << "所在城市\t";
cout << "预定人数" << endl;
while (1)
{
cin >> nickname >> contact >> city >> n;
if (current_number + n <= capacity)
{
Info in(nickname, contact, city, n);
v.push_back(in);
current_number += n;
}
else
{
cout << "对不起,只剩" << capacity - current_number << "个位置.\n";
cout << "1.输入u,更新(updata)预定信息" << endl;
cout << "2.输入q,退出预定" << endl;
cout << "你的选择:";
char c;
cin >> c;
if (c == 'q') break;
else if (c == 'u') continue;
else break;
}
if (current_number == capacity) break;
}
cout << endl;
cout << "截至目前,一共有" << current_number<< "位听众预定参加.";
if (current_number!= 0)
{
cout << "预定听众信息如下:" << endl;
for (auto i = 0; i < v.size(); i++)
{
v[i].print();
cout << endl;
}
}
}
运行结果
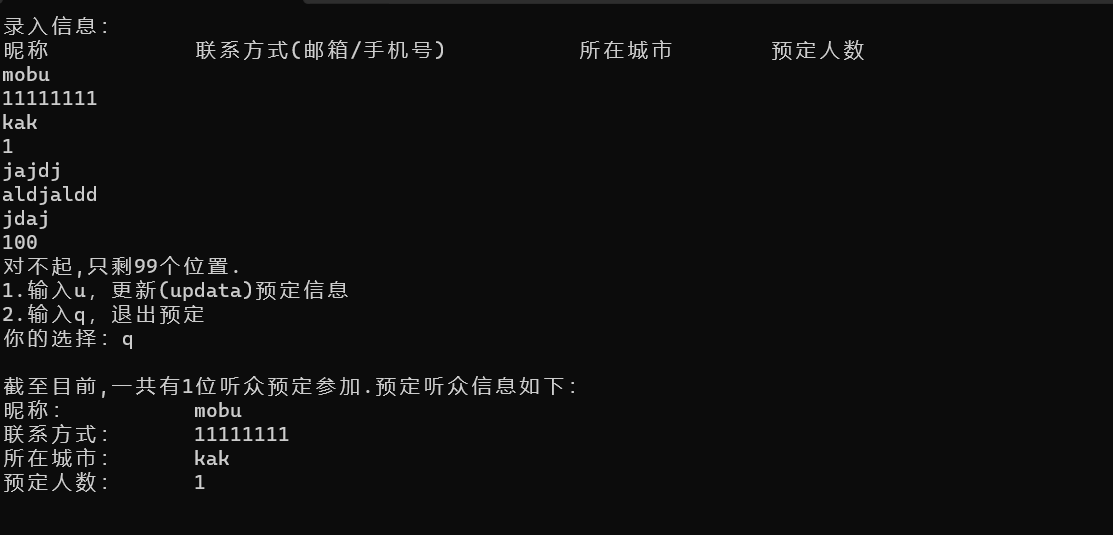