4.6 Checkbox(复选框)
2.CheckBox (复选框)
如题,复选框,即可以同时选中多个选项,至于获得选中的值,同样有两种方式:
1.为每个CheckBox添加事件:setOnCheckedChangeListener
2.弄一个按钮,在点击后,对每个checkbox进行判断:isChecked();
check_box.xml:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/linerLayout1" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="请选择水果:" android:textSize="30sp"/> <CheckBox android:id="@+id/banana" android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="10dp" android:text="香蕉?" android:textSize="30sp"> </CheckBox> <CheckBox android:id="@+id/apple" android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="10dp" android:text="苹果?" android:textSize="30sp"> </CheckBox> <CheckBox android:id="@+id/strawberry" android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="10dp" android:text="草莓?" android:textSize="30sp"> </CheckBox> <Button android:id="@+id/btnpost" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="提交" android:textSize="30sp"/> </LinearLayout>
MainActivity.java:
package com.example.myapplication; import androidx.appcompat.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.CheckBox; import android.widget.CompoundButton; import android.widget.RadioGroup; import android.widget.Toast; public class MainActivity extends AppCompatActivity implements View.OnClickListener,CompoundButton.OnCheckedChangeListener { private CheckBox cb1; private CheckBox cb2; private CheckBox cb3; private Button btn_send; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.checkbox); cb1 = (CheckBox) findViewById(R.id.banana); cb2 = (CheckBox) findViewById(R.id.apple); cb3 = (CheckBox) findViewById(R.id.strawberry); btn_send = (Button) findViewById(R.id.btnpost); cb1.setOnCheckedChangeListener(this); cb2.setOnCheckedChangeListener(this); cb3.setOnCheckedChangeListener(this); btn_send.setOnClickListener(this); } @Override public void onCheckedChanged(CompoundButton compoundButton,boolean b){ if(compoundButton.isChecked()) Toast.makeText(this,compoundButton.getText().toString(),Toast.LENGTH_LONG).show(); } @Override public void onClick(View view){ String choose = " "; if(cb1.isChecked()) choose += cb1.getText().toString() + " "; if (cb2.isChecked()) choose += cb2.getText().toString() + " "; if(cb3.isChecked()) choose += cb3.getText().toString() + " "; Toast.makeText(this,choose,Toast.LENGTH_LONG).show(); } }
进行运行测试:
选中香蕉?/苹果?/草莓?:
提交:
- 自定义点击效果:
在res下的drawable文件夹下新建checkbox.xml文件。
<?xml version="1.0" encoding="utf-8"?> <selector xmlns:android="http://schemas.android.com/apk/res/android"> <item android:state_enabled="true" android:state_checked="true" android:drawable="@mipmap/weixin"/> <item android:state_enabled="true" android:state_checked="false" android:drawable="@mipmap/wode"/> </selector>
写好后,我们有两种方法设置,也可以说一种吧!你看看就知道了~
①android:button属性设置为上述的selctor
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/linerLayout1" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="请选择水果:" android:textSize="30sp"/> <CheckBox android:id="@+id/banana" android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="10dp" android:text="香蕉?" android:button="@drawable/checkbox" android:textSize="30sp"> </CheckBox> <CheckBox android:id="@+id/apple" android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="10dp" android:text="苹果?" android:textSize="30sp"> </CheckBox> <CheckBox android:id="@+id/strawberry" android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="10dp" android:text="草莓?" android:textSize="30sp"> </CheckBox> <Button android:id="@+id/btnpost2" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="提交" android:textSize="30sp" /> </LinearLayout>
②在style中定义一个属性,然后通过android style属性设置,先往style添加下述代码:
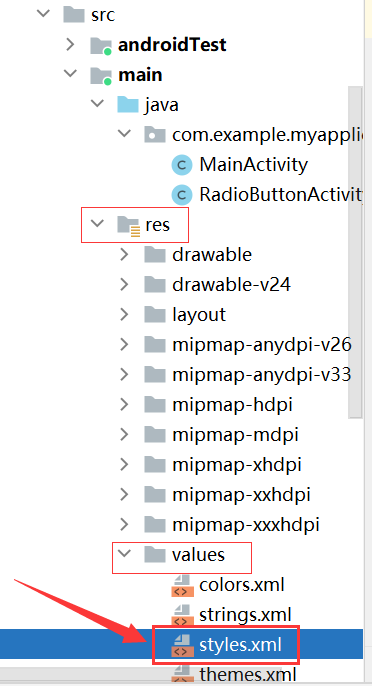
<?xml version="1.0" encoding="utf-8"?> <resources> <style name="checkbox" parent="Widget.AppCompat.CompoundButton.CheckBox"> <item name="android:button">@drawable/checkbox</item> </style> </resources>
然后布局那里:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/linerLayout1" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="请选择水果:" android:textSize="30sp"/> <CheckBox android:id="@+id/banana" android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="10dp" android:text="香蕉?" android:button="@drawable/checkbox" android:textSize="30sp"> </CheckBox> <CheckBox android:id="@+id/apple" android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="10dp" android:text="苹果?" android:textSize="30sp" style="@style/checkbox"> </CheckBox> <CheckBox android:id="@+id/strawberry" android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="10dp" android:text="草莓?" android:textSize="30sp"> </CheckBox> <Button android:id="@+id/btnpost2" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="提交" android:textSize="30sp" /> </LinearLayout>
- 修改文字与选择框的距离
android:background="@null"
android:paddingLeft="100dp"
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/linerLayout1" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="请选择水果:" android:textSize="30sp"/> <CheckBox android:id="@+id/banana" android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="10dp" android:text="香蕉?" android:button="@drawable/checkbox" android:textSize="30sp"> </CheckBox> <CheckBox android:id="@+id/apple" android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="10dp" android:text="苹果?" android:textSize="30sp" style="@style/checkbox"> </CheckBox> <CheckBox android:id="@+id/strawberry" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="草莓?" android:textSize="30sp" android:background="@null" android:paddingLeft="100dp"> </CheckBox> <Button android:id="@+id/btnpost2" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="提交" android:textSize="30sp" /> </LinearLayout>