基于华为鲲鹏云服务器CentOS中(或Ubuntu),使用Linux Socket实现:
1. time服务器的客户端服务器,提交程序运行截图
3. 服务器部署到华为云服务器,客户端用Ubuntu虚拟机。
time服务器代码(tms.c)
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <time.h>
#include <sys/socket.h>
#include <netinet/in.h>
#define PORT 8080
int main() {
int server_fd, new_socket;
struct sockaddr_in address;
int addrlen = sizeof(address);
char buffer[1024] = {0};
time_t current_time;
struct tm * time_info;
char timeString[40]; // space to hold the time string
// Creating socket file descriptor
if ((server_fd = socket(AF_INET, SOCK_STREAM, 0)) == 0) {
perror("socket failed");
exit(EXIT_FAILURE);
}
// Setting up the address structure
address.sin_family = AF_INET;
address.sin_addr.s_addr = INADDR_ANY;
address.sin_port = htons(PORT);
// Bind the socket to the address
if (bind(server_fd, (struct sockaddr *)&address, sizeof(address)) < 0) {
perror("bind failed");
exit(EXIT_FAILURE);
}
// Listen for connections
if (listen(server_fd, 3) < 0) {
perror("listen");
exit(EXIT_FAILURE);
}
// Accept a connection
if ((new_socket = accept(server_fd, (struct sockaddr *)&address, (socklen_t*)&addrlen)) < 0) {
perror("accept");
exit(EXIT_FAILURE);
}
// Get the current time and format it
time(¤t_time);
time_info = localtime(¤t_time);
strftime(timeString, sizeof(timeString), "%Y-%m-%d %H:%M:%S", time_info);
// Send the time to the client
send(new_socket, timeString, strlen(timeString), 0);
printf("Time sent to client\n");
return 0;
}
time客户端代码(tmc.c)
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#define PORT 8080
int main() {
int sock = 0;
struct sockaddr_in serv_addr;
char buffer[1024] = {0};
if ((sock = socket(AF_INET, SOCK_STREAM, 0)) < 0) {
printf("\n Socket creation error \n");
return -1;
}
serv_addr.sin_family = AF_INET;
serv_addr.sin_port = htons(PORT);
// Convert IPv4 and IPv6 addresses from text to binary form
if(inet_pton(AF_INET, "127.0.0.1", &serv_addr.sin_addr) <= 0) {
printf("\nInvalid address/ Address not supported \n");
return -1;
}
if (connect(sock, (struct sockaddr *)&serv_addr, sizeof(serv_addr)) < 0) {
printf("\nConnection Failed \n");
return -1;
}
read(sock, buffer, 1024);
printf("Time from server: %s\n", buffer);
return 0;
}
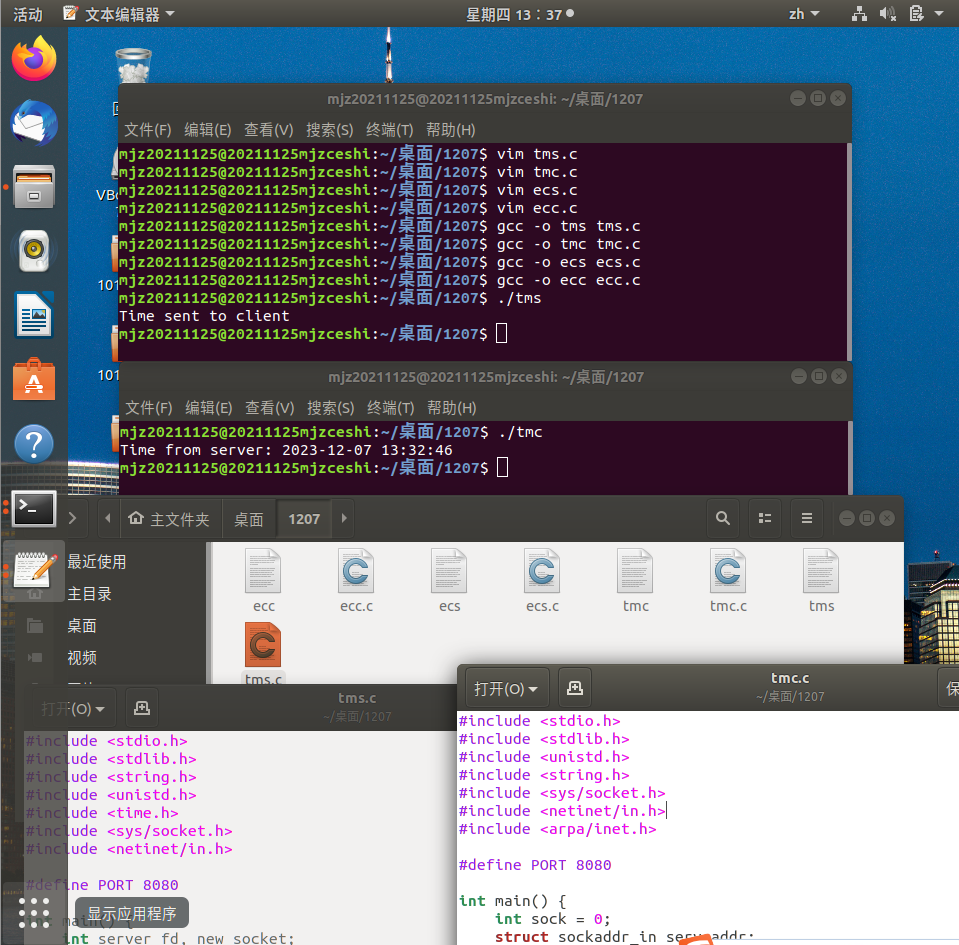
2. echo服务器的客户端服务器,提交程序运行截图,服务器把客户端传进来的内容加入“服务器进程pid 你的学号 姓名 echo :”返回给客户端
echo服务器代码(ecs.c)
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <sys/socket.h>
#include <netinet/in.h>
#define PORT 8081
int main() {
int server_fd, new_socket;
struct sockaddr_in address;
int addrlen = sizeof(address);
char buffer[1024] = {0};
char response[1024];
// Creating socket file descriptor
if ((server_fd = socket(AF_INET, SOCK_STREAM, 0)) == 0) {
perror("socket failed");
exit(EXIT_FAILURE);
}
// Setting up the address structure
address.sin_family = AF_INET;
address.sin_addr.s_addr = INADDR_ANY;
address.sin_port = htons(PORT);
// Bind the socket to the address
if (bind(server_fd, (struct sockaddr *)&address, sizeof(address)) < 0) {
perror("bind failed");
exit(EXIT_FAILURE);
}
// Listen for connections
if (listen(server_fd, 3) < 0) {
perror("listen");
exit(EXIT_FAILURE);
}
// Accept a connection
if ((new_socket = accept(server_fd, (struct sockaddr *)&address, (socklen_t*)&addrlen)) < 0) {
perror("accept");
exit(EXIT_FAILURE);
}
// Read the message from client
read(new_socket, buffer, 1024);
printf("Received from client: %s\n", buffer);
// Prepare the response
sprintf(response, "Server PID %d 20211125 苗靖章 echo: %s", getpid(), buffer);
// Send the response back to client
send(new_socket, response, strlen(response), 0);
printf("Response sent to client\n");
return 0;
}
echo客户端代码(ecc.c)
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#define PORT 8081
int main() {
int sock = 0;
struct sockaddr_in serv_addr;
char buffer[1024] = {0};
char *message = "Hello from client";
if ((sock = socket(AF_INET, SOCK_STREAM, 0)) < 0) {
printf("\n Socket creation error \n");
return -1;
}
serv_addr.sin_family = AF_INET;
serv_addr.sin_port = htons(PORT);
// Convert IPv4 and IPv6 addresses from text to binary form
if(inet_pton(AF_INET, "127.0.0.1", &serv_addr.sin_addr) <= 0) {
printf("\nInvalid address/ Address not supported \n");
return -1;
}
if (connect(sock, (struct sockaddr *)&serv_addr, sizeof(serv_addr)) < 0) {
printf("\nConnection Failed \n");
return -1;
}
send(sock, message, strlen(message), 0);
printf("Message sent to server\n");
read(sock, buffer, 1024);
printf("Response from server: %s\n", buffer);
return 0;
}
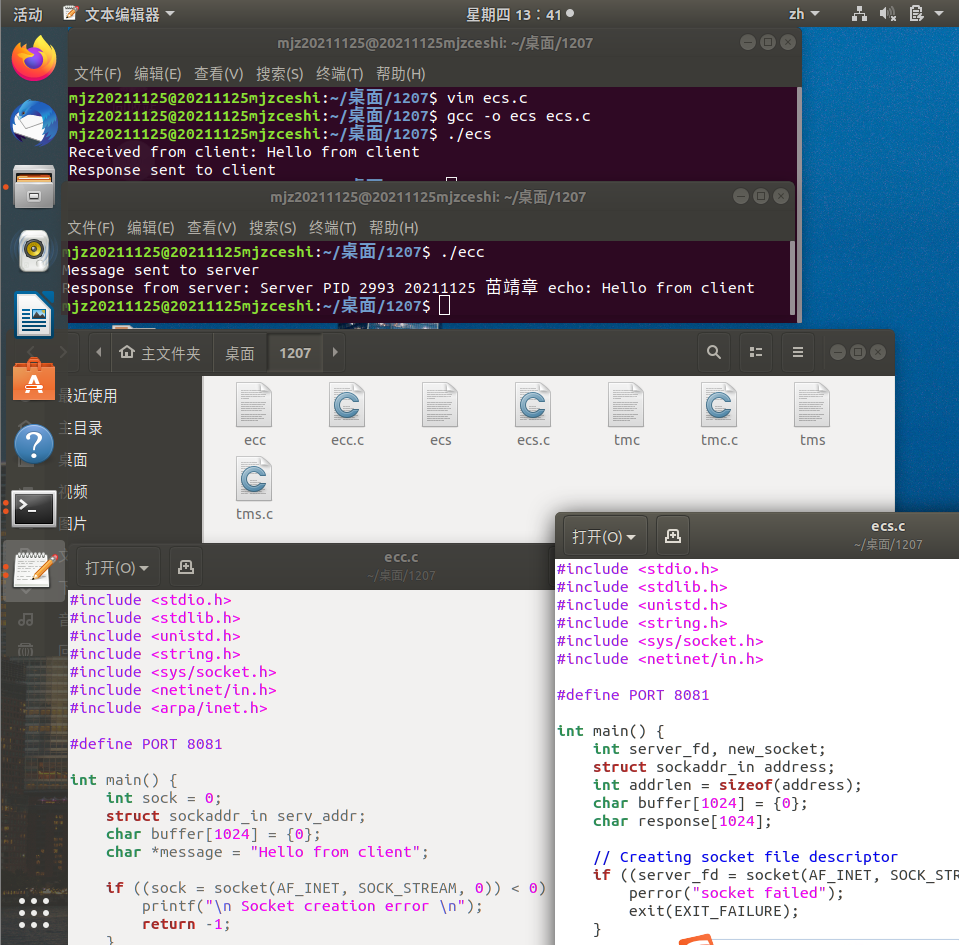
4. 要用多线程或者多进程实现,至少连接两个客户端。
更新echo代码
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <pthread.h>
#define PORT 8081
#define MAX_CLIENTS 10
void *client_handler(void *socket_desc) {
int sock = *(int*)socket_desc;
int read_size;
char client_message[1024];
char response[1024];
// Read the message from client
while ((read_size = recv(sock, client_message, sizeof(client_message), 0)) > 0) {
client_message[read_size] = '\0'; // Null-terminate the string
// Prepare the response
sprintf(response, "Server PID %d 20211125 苗靖章 echo: %s", getpid(), client_message);
// Send the response back to client
send(sock, response, strlen(response), 0);
}
if (read_size == 0) {
puts("Client disconnected");
} else if (read_size == -1) {
perror("recv failed");
}
free(socket_desc);
return 0;
}
int main() {
int server_fd, client_sock, *new_sock;
struct sockaddr_in server, client;
int c = sizeof(struct sockaddr_in);
// Create socket
server_fd = socket(AF_INET, SOCK_STREAM, 0);
if (server_fd == -1) {
perror("Could not create socket");
return 1;
}
// Prepare the sockaddr_in structure
server.sin_family = AF_INET;
server.sin_addr.s_addr = INADDR_ANY;
server.sin_port = htons(PORT);
// Bind
if (bind(server_fd, (struct sockaddr *)&server, sizeof(server)) < 0) {
perror("bind failed");
return 1;
}
// Listen
listen(server_fd, MAX_CLIENTS);
// Accept incoming connections
puts("Waiting for incoming connections...");
while ((client_sock = accept(server_fd, (struct sockaddr *)&client, (socklen_t*)&c))) {
puts("Connection accepted");
pthread_t sniffer_thread;
new_sock = malloc(1);
*new_sock = client_sock;
if (pthread_create(&sniffer_thread, NULL, client_handler, (void*) new_sock) < 0) {
perror("could not create thread");
return 1;
}
// Optionally, you can detach the thread for concurrent handling
// pthread_detach(sniffer_thread);
}
if (client_sock < 0) {
perror("accept failed");
return 1;
}
return 0;
}
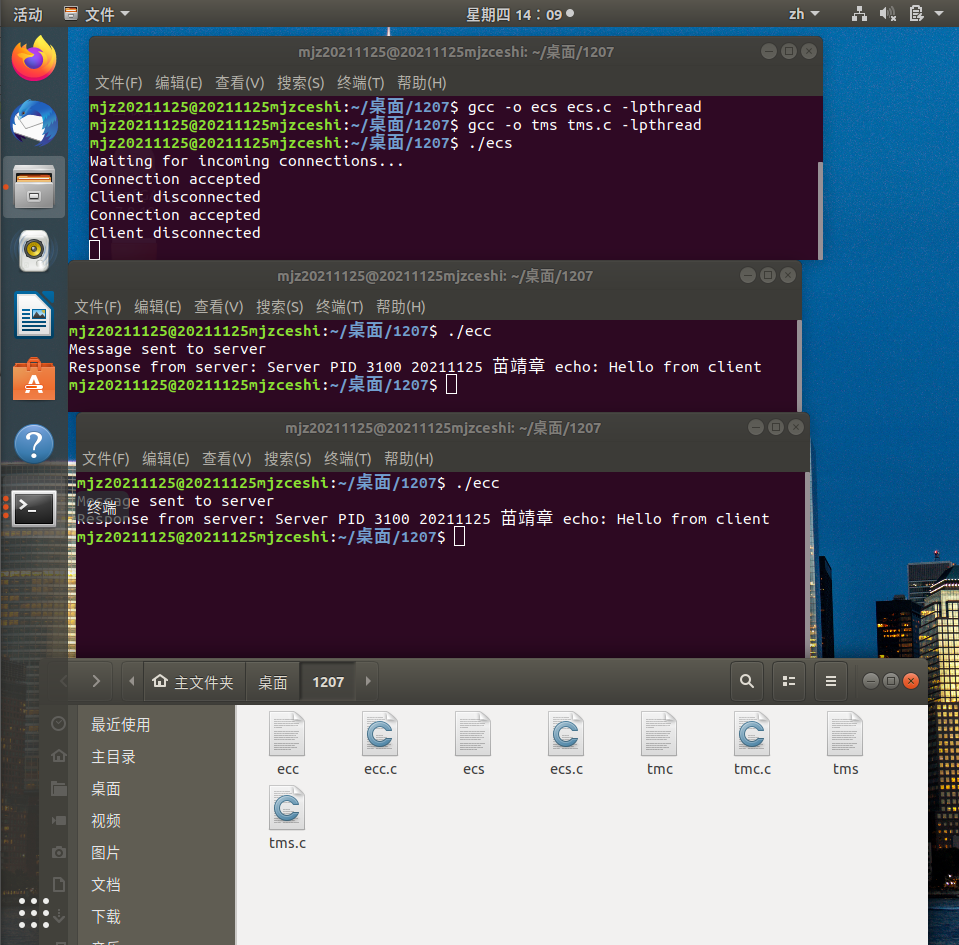
更新time代码:
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <time.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <pthread.h>
#define PORT 8080
void *client_handler(void *socket_desc) {
int sock = *(int*)socket_desc;
time_t current_time;
struct tm * time_info;
char timeString[40]; // space to hold the time string
// Get the current time and format it
time(¤t_time);
time_info = localtime(¤t_time);
strftime(timeString, sizeof(timeString), "%Y-%m-%d %H:%M:%S", time_info);
// Send the time to the client
write(sock, timeString, strlen(timeString));
printf("Time sent to client\n");
close(sock);
free(socket_desc);
return 0;
}
int main() {
int server_fd, client_sock, *new_sock;
struct sockaddr_in server, client;
int c = sizeof(struct sockaddr_in);
// Create socket
server_fd = socket(AF_INET, SOCK_STREAM, 0);
if (server_fd == -1) {
perror("Could not create socket");
return 1;
}
// Prepare the sockaddr_in structure
server.sin_family = AF_INET;
server.sin_addr.s_addr = INADDR_ANY;
server.sin_port = htons(PORT);
// Bind
if (bind(server_fd, (struct sockaddr *)&server, sizeof(server)) < 0) {
perror("bind failed");
return 1;
}
// Listen
listen(server_fd, 3);
// Accept incoming connections
puts("Waiting for incoming connections...");
while ((client_sock = accept(server_fd, (struct sockaddr *)&client, (socklen_t*)&c))) {
puts("Connection accepted");
pthread_t sniffer_thread;
new_sock = malloc(1);
*new_sock = client_sock;
if (pthread_create(&sniffer_thread, NULL, client_handler, (void*) new_sock) < 0) {
perror("could not create thread");
return 1;
}
// Optionally, you can detach the thread for concurrent handling
// pthread_detach(sniffer_thread);
}
if (client_sock < 0) {
perror("accept failed");
return 1;
}
return 0;
}
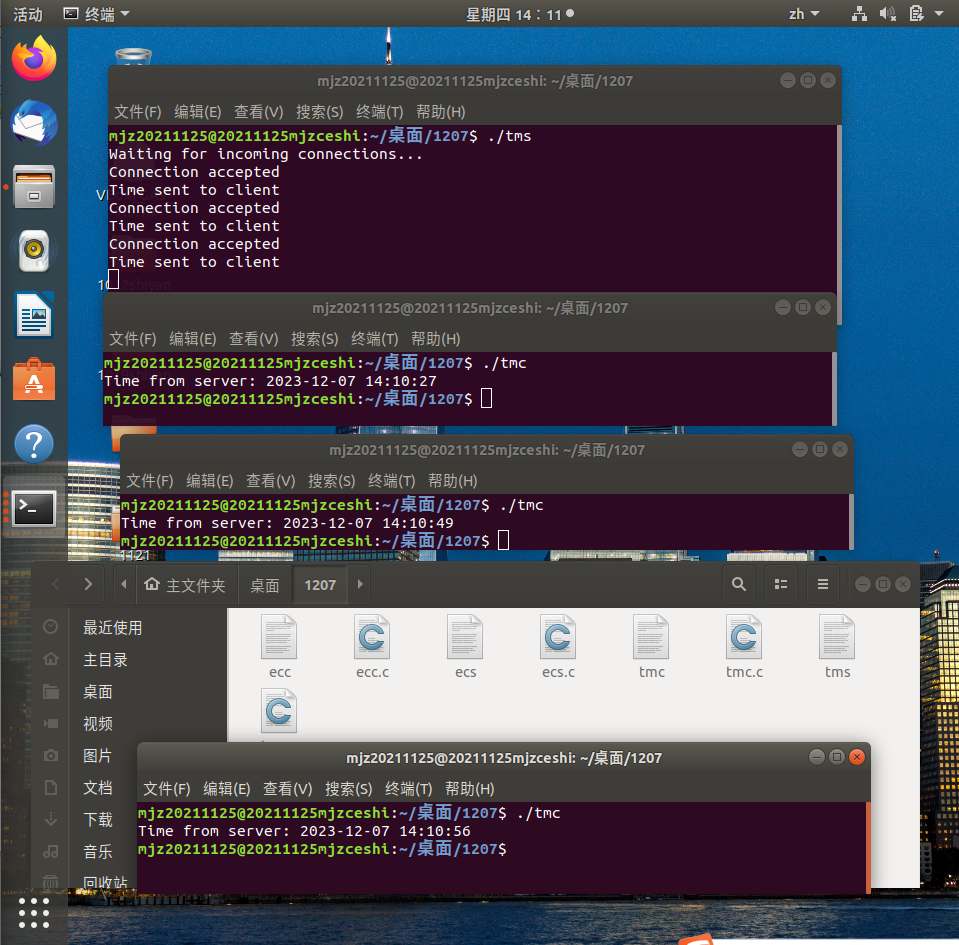
5. 把服务器部署到试验箱。(加分项)