2023/9/6 09:05:14
要通过HTML、JavaScript和CSS实现点击菜单栏后瞄点到相应位置,可以按照以下步骤进行操作:
- HTML结构: 在HTML中,定义一个菜单栏和对应的内容区域,例如:
<nav>
<ul>
<li><a href="#section1">Section 1</a></li>
<li><a href="#section2">Section 2</a></li>
<li><a href="#section3">Section 3</a></li>
</ul>
</nav>
<section id="section1">
<h2>Section 1</h2>
<!-- Section 1 的内容 -->
</section>
<section id="section2">
<h2>Section 2</h2>
<!-- Section 2 的内容 -->
</section>
<section id="section3">
<h2>Section 3</h2>
<!-- Section 3 的内容 -->
</section>
- CSS样式: 使用CSS样式美化菜单栏,使其具有点击效果,以及其他需要的样式。例如:
nav ul {
list-style-type: none;
margin: 0;
padding: 0;
}
nav ul li {
display: inline-block;
margin-right: 10px;
}
nav ul li a {
text-decoration: none;
color: #000;
padding: 5px;
border-radius: 5px;
background-color: #ccc;
transition: background-color 0.3s;
}
nav ul li a:hover {
background-color: #aaa;
}
- JavaScript交互: 使用JavaScript添加点击事件,当点击菜单栏时,页面滚动到相应位置。例如:
// 获取菜单栏中的所有链接
const menuLinks = document.querySelectorAll('nav ul li a');
// 遍历每个链接,添加点击事件
for (let link of menuLinks) {
link.addEventListener('click', (event) => {
event.preventDefault(); // 阻止默认的链接跳转行为
const targetId = link.getAttribute('href'); // 获取链接的目标位置id
const targetElement = document.querySelector(targetId); // 获取目标元素
// 使用scrollIntoView方法将页面滚动到目标元素位置
targetElement.scrollIntoView({ behavior: 'smooth' });
});
}
以上代码通过获取菜单栏中的链接,并为每个链接添加点击事件,在点击时阻止默认的链接跳转行为,并使用scrollIntoView
方法将页面平滑滚动到目标元素的位置。
通过这样的实现,当用户点击菜单栏中的链接时,页面会平滑地滚动到相应的区域位置。
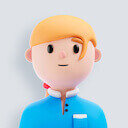
2023/9/6 09:06:17
2023/9/6 09:07:12
如果您在使用Vue 3 + Vite + TypeScript来实现点击菜单栏后瞄点到相应位置,可以按照以下步骤进行操作:
- 创建一个Vue 3项目: 首先,使用Vite快速创建一个Vue 3项目。打开命令行终端,执行以下命令:
npm init vite@latest my-app --template vue-ts
cd my-app
npm install
- 编辑组件文件: 在
src/components
目录下创建一个名为Menu.vue
的组件文件,并编辑该文件:
<template>
<nav>
<ul>
<li v-for="(section, index) in sections" :key="index">
<a :href="`#${section.id}`" @click="scrollToSection(section.id)">
{{ section.name }}
</a>
</li>
</ul>
</nav>
</template>
<script lang="ts">
import { defineComponent } from 'vue';
export default defineComponent({
name: 'Menu',
data() {
return {
sections: [
{ id: 'section1', name: 'Section 1' },
{ id: 'section2', name: 'Section 2' },
{ id: 'section3', name: 'Section 3' }
]
};
},
methods: {
scrollToSection(id: string) {
const targetElement = document.querySelector(id);
if (targetElement) {
targetElement.scrollIntoView({ behavior: 'smooth' });
}
}
}
});
</script>
<style scoped>
nav ul {
list-style-type: none;
margin: 0;
padding: 0;
}
nav ul li {
display: inline-block;
margin-right: 10px;
}
nav ul li a {
text-decoration: none;
color: #000;
padding: 5px;
border-radius: 5px;
background-color: #ccc;
transition: background-color 0.3s;
}
nav ul li a:hover {
background-color: #aaa;
}
</style>
在上述代码中,我们创建了一个名为Menu
的Vue组件。该组件渲染了一个菜单栏,使用v-for
指令循环渲染菜单项。每个菜单项绑定了一个点击事件scrollToSection
,用于滚动到相应的区域。
- 在主应用中使用组件: 在App.vue文件中,使用
Menu
组件来展示菜单栏。
<template>
<div>
<Menu />
<main>
<section id="section1">
<h2>Section 1</h2>
<!-- Section 1的内容 -->
</section>
<section id="section2">
<h2>Section 2</h2>
<!-- Section 2的内容 -->
</section>
<section id="section3">
<h2>Section 3</h2>
<!-- Section 3的内容 -->
</section>
</main>
</div>
</template>
<script lang="ts">
import Menu from './components/Menu.vue';
import { defineComponent } from 'vue';
export default defineComponent({
name: 'App',
components: {
Menu
}
});
</script>
在主应用的模板中,我们引入了Menu
组件,并在之后定义了三个区域(Section)。当用户点击菜单栏时,会自动平滑滚动到相应的区域。
通过以上步骤,您就可以在Vue 3 + Vite + TypeScript项目中实现点击菜单栏后瞄点到相应位置的功能。
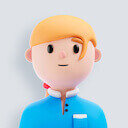
2023/9/6 09:11:02
2023/9/6 09:11:24
如果您不想使用v-for
循环遍历菜单项,可以手动创建菜单项,并为每个菜单项添加点击事件。以下是相应的代码示例:
<template>
<nav>
<ul>
<li>
<a href="#section1" @click="scrollToSection('section1')">Section 1</a>
</li>
<li>
<a href="#section2" @click="scrollToSection('section2')">Section 2</a>
</li>
<li>
<a href="#section3" @click="scrollToSection('section3')">Section 3</a>
</li>
</ul>
</nav>
</template>
<script lang="ts">
import { defineComponent } from 'vue';
export default defineComponent({
name: 'Menu',
methods: {
scrollToSection(id: string) {
const targetElement = document.querySelector(id);
if (targetElement) {
targetElement.scrollIntoView({ behavior: 'smooth' });
}
}
}
});
</script>
<style scoped>
nav ul {
list-style-type: none;
margin: 0;
padding: 0;
}
nav ul li {
display: inline-block;
margin-right: 10px;
}
nav ul li a {
text-decoration: none;
color: #000;
padding: 5px;
border-radius: 5px;
background-color: #ccc;
transition: background-color 0.3s;
}
nav ul li a:hover {
background-color: #aaa;
}
</style>
在上述代码中,我们手动创建了菜单项,并为每个菜单项添加了点击事件scrollToSection
。在点击菜单项时,会触发该事件,进行平滑滚动到相应的区域。
注意:为了保持代码简洁性,我们在这里直接使用了固定的菜单项,如果菜单项过多或需要从数据源中动态生成,您可能需要通过循环或其他方式来生成菜单项。不使用v-for
只是针对固定菜单项的情况下的一种实现方式。
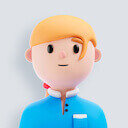
2023/9/6 09:27:19
2023/9/6 09:27:33
这段代码的作用是检查目标元素是否存在,如果存在则对目标元素执行平滑滚动到可视区域的操作。
首先,document.querySelector(id)
用于查找具有指定id的元素。如果找到了匹配的元素,返回该元素的引用;如果没有找到匹配的元素,则返回null
。
接下来,通过条件判断if (targetElement)
来确保目标元素存在。如果目标元素存在,则调用scrollIntoView()
方法来实现平滑滚动。scrollIntoView()
方法会使目标元素滚动到可视区域内,并且可以通过behavior: 'smooth'
参数来指定滚动的行为为平滑滚动,即带有动画效果的滚动。
简而言之,这段代码的目的是在点击菜单项后,通过滚动将相应的内容区域平滑滚动到可视区域内,以提供更好的用户体验。