Java读取两个txt内容并作集合运算
文件内容及格式
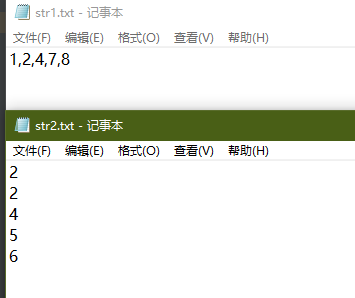
完整代码
package com.example.fortotest;
import java.io.BufferedReader;
import java.io.File;
import java.io.InputStreamReader;
import java.nio.file.Files;
import java.util.HashSet;
import java.util.Objects;
public class Day3 {
public static final String SPLIT_SYMBOL = ",";
public static void main(String[] args) {
try {
// 将st1记为集合A str2文件记为集合B
// 逗号连接的数字字符串
String file1 = "E:\\str1.txt";
File filePath1 = new File(file1);
HashSet<Long> hashSet1 = new HashSet<>();
BufferedReader bufferedReader1 = new BufferedReader(new InputStreamReader(Files.newInputStream(filePath1.toPath())));
String str1;
while ((str1 = bufferedReader1.readLine()) != null) {
String[] split = str1.split(SPLIT_SYMBOL);
for (String s : split) {
hashSet1.add(Long.parseLong(s.trim()));
}
}
// 以行划分的数字字符串
String file2 = "E:\\str2.txt";
File filePath2 = new File(file2);
// 交集
HashSet<Long> intersection = new HashSet<>();
// 并集
HashSet<Long> unionSet = new HashSet<>(hashSet1);
// str2与str1的差集
HashSet<Long> str2AndStr1DifferenceSet = new HashSet<>();
String str2;
BufferedReader bufferedReader2 = new BufferedReader(new InputStreamReader(Files.newInputStream(filePath2.toPath())));
while (!Objects.equals(str2 = bufferedReader2.readLine(), "") && str2 != null) {
long l = Long.parseLong(str2.trim());
if (hashSet1.contains(l)) {
// 交集
intersection.add(l);
} else {
// 并集
unionSet.add(l);
// A对B的差集
str2AndStr1DifferenceSet.add(l);
}
}
// 对称差集
HashSet<Long> symmetricDifferenceSet = new HashSet<>(unionSet);
symmetricDifferenceSet.removeAll(intersection);
// str2对str1的差集
HashSet<Long> str1AndStr2DifferenceSet = new HashSet<>(symmetricDifferenceSet);
str1AndStr2DifferenceSet.removeAll(str2AndStr1DifferenceSet);
System.out.printf("A∩B(交集) : %s%n", intersection);
System.out.printf("A∪B(并集) : %s%n", unionSet);
System.out.printf("A−B(str1与str2的差集) : %s%n", str1AndStr2DifferenceSet);
System.out.printf("B-A(str2与str1的差集) : %s%n", str2AndStr1DifferenceSet);
System.out.printf("A⊕B(对称差集) : %s%n", symmetricDifferenceSet);
HashSet<Long> a = new HashSet<>(intersection);
HashSet<Long> b = new HashSet<>(intersection);
a.addAll(str1AndStr2DifferenceSet);
b.addAll(str2AndStr1DifferenceSet);
System.out.printf("集合A去重后 : %s%n", a);
System.out.printf("集合B去重后 : %s%n", b);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
执行结果
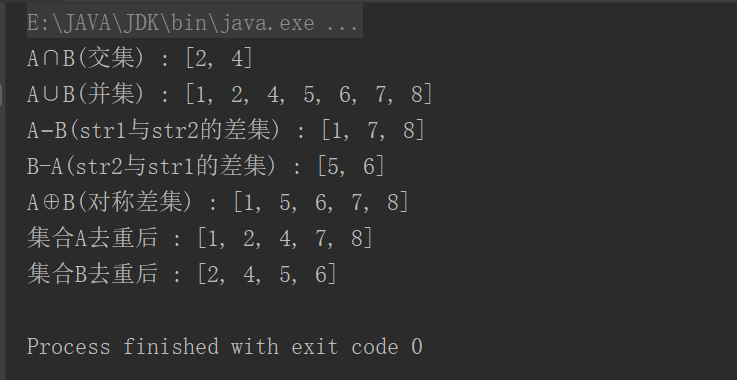