8. (简答题) 请完善课上的口算题卡代码,实现重复题目的检测、题目数字范围、加减乘除算式的参数化等扩展功能,提交代码和运行截图。
package org.example;
import java.util.Random;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入题目的最小数字范围:");
int minRange = scanner.nextInt();
System.out.print("请输入题目的最大数字范围:");
int maxRange = scanner.nextInt();
System.out.print("请输入生成题目的数量:");
int numberOfQuestions = scanner.nextInt();
System.out.print("请选择算式类型(1: 加法, 2: 减法, 3: 乘法, 4: 除法):");
int operatorChoice = scanner.nextInt();
System.out.print("请输入每个算式中的操作数数量:");
int operandCount = scanner.nextInt();
scanner.close();
printArithmeticQuestions(minRange, maxRange, numberOfQuestions, operatorChoice, operandCount);
}
public static void printArithmeticQuestions(int minRange, int maxRange, int numberOfQuestions, int operatorChoice, int operandCount) {
System.out.println("生成的口算题目如下:\n");
Random random = new Random();
int questionsPerLine = 5; // 每行显示的题目数量
for (int i = 1; i <= numberOfQuestions; i++) {
String question = generateRandomQuestion(minRange, maxRange, operatorChoice, operandCount);
System.out.printf(question + "\t");
// 每行显示指定数量的题目后,换行
if (i % questionsPerLine == 0) {
System.out.println(); // 换行
}
}
}
public static String generateRandomQuestion(int minRange, int maxRange, int operatorChoice, int operandCount) {
Random random = new Random();
StringBuilder question = new StringBuilder();
for (int i = 0; i < operandCount; i++) {
int operand = random.nextInt(maxRange - minRange + 1) + minRange;
question.append(operand);
if (i < operandCount - 1) {
question.append(" ");
question.append(getOperatorSymbol(operatorChoice));
question.append(" ");
}
}
question.append(" = ");
return question.toString();
}
public static String getOperatorSymbol(int operatorChoice) {
switch (operatorChoice) {
case 1:
return "+";
case 2:
return "-";
case 3:
return "×";
case 4:
return "÷";
default:
return "";
}
}
}
运行结果示意图:
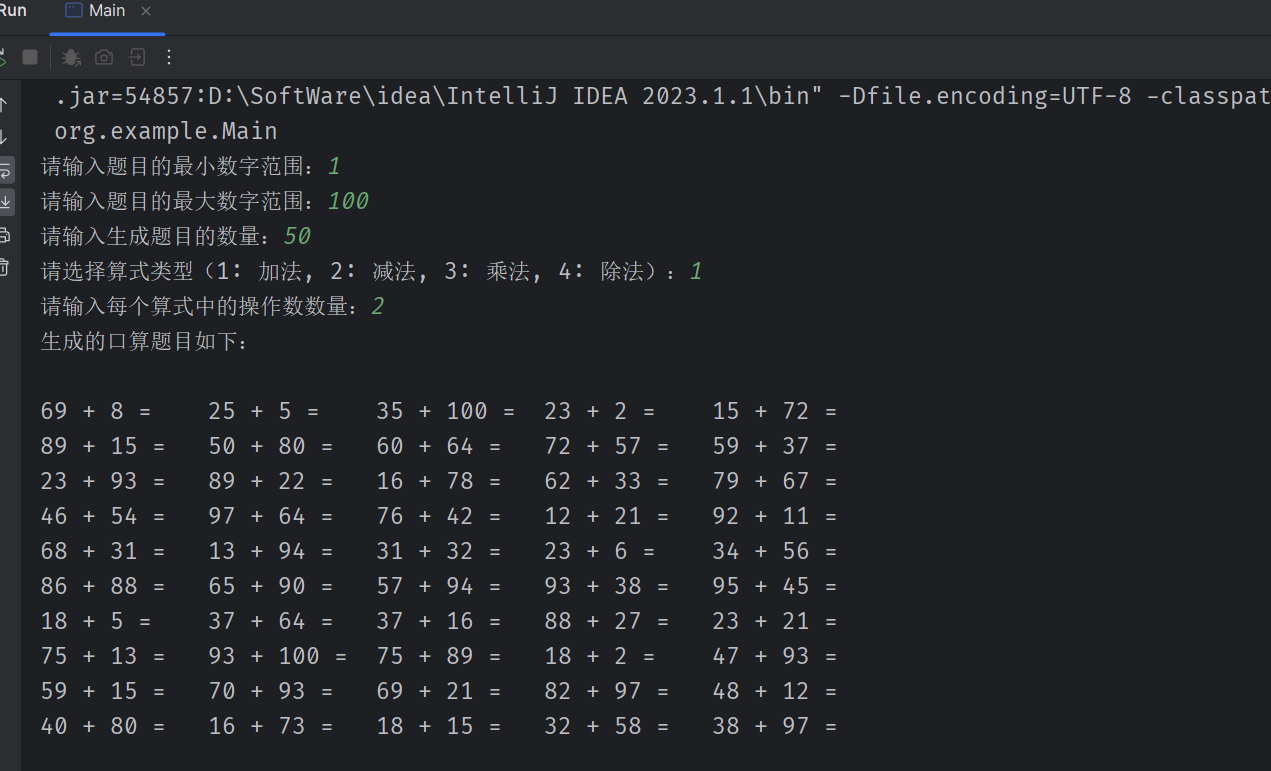
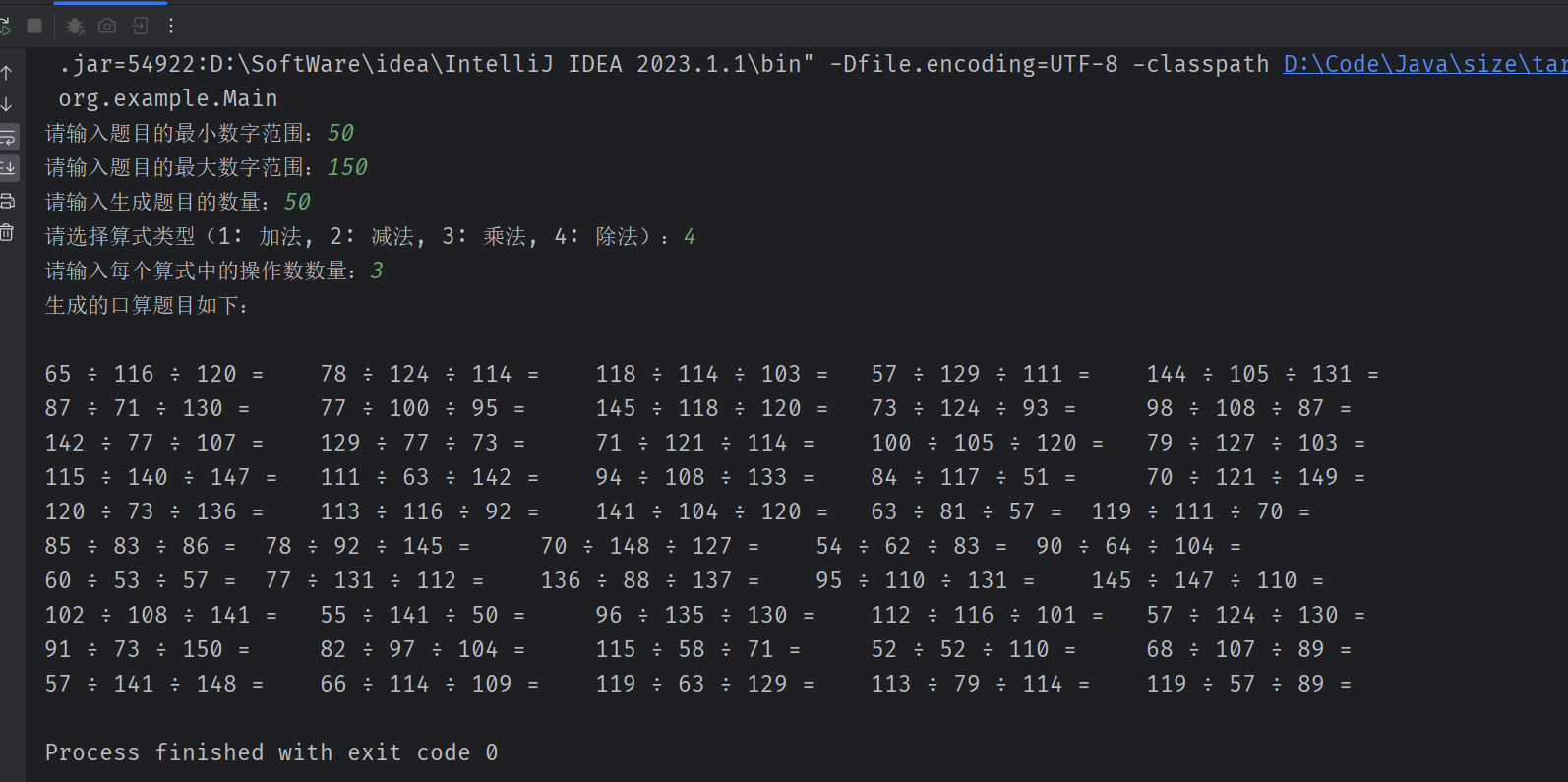