DES对称加密算法Java实现
源代码AESUtils.java
//package me.muphy.util;
import javax.crypto.*;
import javax.crypto.spec.SecretKeySpec;
import java.nio.charset.StandardCharsets;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.security.SecureRandom;
import java.util.Base64;
public class AESUtils {
private static final String AES = "AES";
private static SecretKey secretKey = null;
public static SecretKey getSecretKey() throws NoSuchAlgorithmException {
if (secretKey != null) {
return secretKey;
}
KeyGenerator keyGenerator = KeyGenerator.getInstance(AES);
keyGenerator.init(new SecureRandom());//不使用种子,每次生成的都不同,可以不要
secretKey = keyGenerator.generateKey();
return secretKey;
}
public static String encrypt(String data) throws InvalidKeyException, NoSuchAlgorithmException, NoSuchPaddingException, IllegalBlockSizeException, BadPaddingException {
SecretKey secretKey = getSecretKey();
return encrypt(data, secretKey);
}
public static String encrypt(String data, String secret) throws InvalidKeyException, NoSuchAlgorithmException, NoSuchPaddingException, IllegalBlockSizeException, BadPaddingException {
SecretKey secretKey = toSecretKey(secret);
return encrypt(data, secretKey);
}
public static String encrypt(String data, SecretKey secretKey) throws InvalidKeyException, NoSuchAlgorithmException, NoSuchPaddingException, IllegalBlockSizeException, BadPaddingException {
Cipher cipher = Cipher.getInstance(AES);
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
byte[] bytes = data.getBytes(StandardCharsets.UTF_8);
byte[] encryptedData = cipher.doFinal(bytes);
return base64Encrypt(encryptedData);
}
public static String decrypt(String data) throws NoSuchAlgorithmException, NoSuchPaddingException, InvalidKeyException, IllegalBlockSizeException, BadPaddingException {
SecretKey secretKey = getSecretKey();
return decrypt(data, secretKey);
}
public static String decrypt(String data, String secret) throws NoSuchAlgorithmException, NoSuchPaddingException, InvalidKeyException, IllegalBlockSizeException, BadPaddingException {
SecretKey secretKey = toSecretKey(secret);
return decrypt(data, secretKey);
}
public static String decrypt(String data, SecretKey secretKey) throws NoSuchAlgorithmException, NoSuchPaddingException, InvalidKeyException, IllegalBlockSizeException, BadPaddingException {
Cipher cipher = Cipher.getInstance(AES);
cipher.init(Cipher.DECRYPT_MODE, secretKey);
byte[] base64Decrypt = base64Decrypt(data);
byte[] decryptedData = cipher.doFinal(base64Decrypt);
return new String(decryptedData, StandardCharsets.UTF_8);
}
private static String base64Encrypt(byte[] bytes) {
byte[] encode = Base64.getEncoder().encode(bytes);
return new String(encode, StandardCharsets.UTF_8);
// return new BASE64Encoder().encode(bytes);
}
private static byte[] base64Decrypt(String data) {
byte[] bytes = data.getBytes(StandardCharsets.UTF_8);
return Base64.getDecoder().decode(bytes);
// return new BASE64Decoder().decodeBuffer(data);
}
private static SecretKey toSecretKey(String secret) {
byte[] decodedKey = Base64.getDecoder().decode(secret);
return new SecretKeySpec(decodedKey, 0, decodedKey.length, "AES");
}
private static String toSecretString(SecretKey secretKey) {
byte[] encoded = secretKey.getEncoded();
return Base64.getEncoder().encodeToString(encoded);
}
public static void main(String[] args) {
try {
String s1 = "hello zian 1";
String es1 = encrypt(s1);
String ds1 = decrypt(es1);
System.out.println(s1 + " = 加密:" + es1 + ",解密:" + ds1);
String s2 = "hello zian 2";
String secret = "yKCzTJcIfnrHVNLR5Xo6zw==";
String es2 = encrypt(s2, secret);
String ds2 = decrypt(es2, secret);
System.out.println(s2 + " = 加密:" + es2 + ",解密:" + ds2);
} catch (NoSuchAlgorithmException | NoSuchPaddingException | InvalidKeyException | IllegalBlockSizeException | BadPaddingException e) {
e.printStackTrace();
}
}
}
测试结果
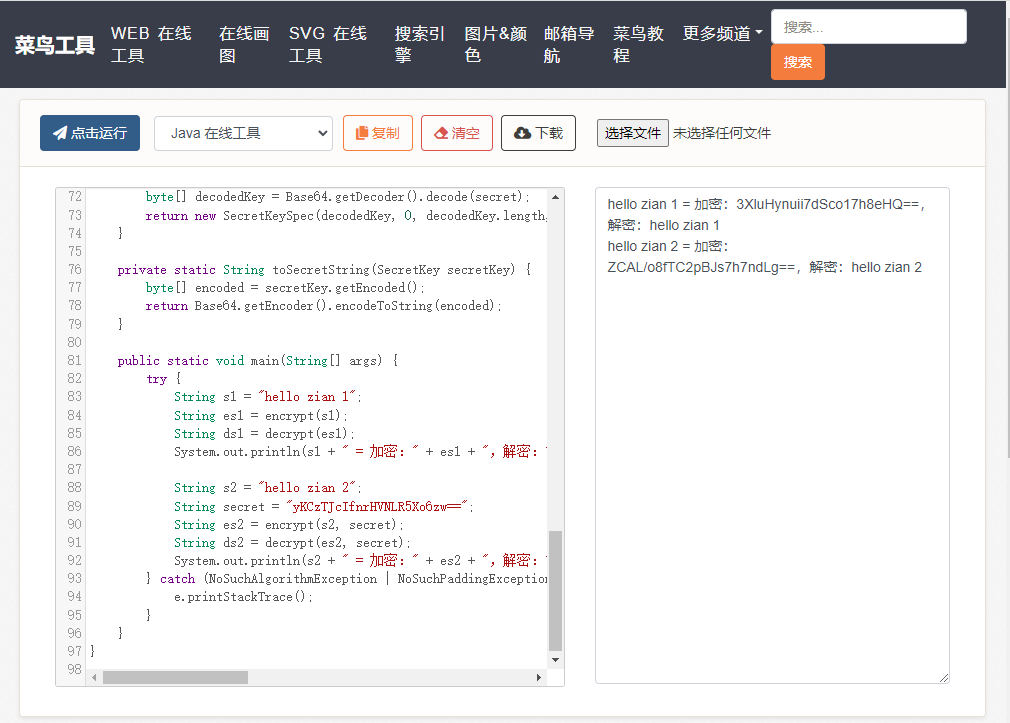