java-httpclient
1.maven
<!-- 主要的jar-->
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.2</version>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.10</version>
</dependency>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.3.2</version>
</dependency>
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.7</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpmime</artifactId>
<version>4.5.2</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.41</version>
</dependency>
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.8</version>
</dependency>
2.实例
2.1 GET
public static String doGet(String url, Map<String, String> params) {
String result = "";
/**
* RequestConfig:
* setSocketTimeout: socket读取数据超时时间
* setConnectTimeout: 与服务器链接超时时间
* setConnectionRequestTimeout: 从连接池获取连接的超时时间
*/
RequestConfig reqConfig = RequestConfig.custom()
.setSocketTimeout(60 * 1000)
.setConnectTimeout(10 * 1000)
.setConnectionRequestTimeout(10 * 1000)
.build();
/**
* HttpClientBuilder:
* setMaxConnTotal: 连接池最大连接数
* setMaxConnPerRoute: 分配给同一个路由最大的并发连接数
* setDefaultConfig(RequestConfig)
*/
HttpClient httpClient = HttpClientBuilder.create()
.setDefaultRequestConfig(reqConfig)
.build();
HttpGet httpGet = null;
try {
URIBuilder uriBuilder = new URIBuilder(url);
if (null != params && !params.isEmpty()) {
for (Map.Entry<String, String> entry : params.entrySet()) {
uriBuilder.addParameter(entry.getKey(), entry.getValue());
}
}
URI uri = uriBuilder.build();
httpGet = new HttpGet(uri);
HttpResponse response = httpClient.execute(httpGet);
if (response.getStatusLine().getStatusCode() == HttpStatus.SC_OK) {
result = EntityUtils.toString(response.getEntity());
log.info("doGetSuccess({}):{}",url, result);
} else {
log.error("doGetFail({}):{}", url, response.getStatusLine().getStatusCode());
}
} catch (Exception e) {
log.error("doGetError({}):{}", url, ExceptionUtils.getStackTrace(e));
} finally {
// 释放连接
if (null != httpGet) {
httpGet.releaseConnection();
}
}
return result;
}
2.2 POST-Common
public static String doPost(String url, Map<String, String> params) {
String result = "";
RequestConfig reqConfig = RequestConfig.custom()
.setSocketTimeout(60 * 1000)
.setConnectTimeout(10 * 1000)
.setConnectionRequestTimeout(10 * 1000)
.build();
/**
* HttpClientBuilder:
* setMaxConnTotal: 连接池最大连接数
* setMaxConnPerRoute: 分配给同一个路由最大的并发连接数
* setDefaultConfig(RequestConfig)
*/
HttpClient httpClient = HttpClientBuilder.create()
.setDefaultRequestConfig(reqConfig)
.build();
HttpPost httpPost = new HttpPost(url);
try { // 参数键值对
if (null != params && !params.isEmpty()) {
List<NameValuePair> pairs = new ArrayList<NameValuePair>();
NameValuePair pair = null;
for (String key : params.keySet()) {
pair = new BasicNameValuePair(key, params.get(key));
pairs.add(pair);
}
// 模拟表单
UrlEncodedFormEntity entity = new UrlEncodedFormEntity(pairs);
httpPost.setEntity(entity);
}
HttpResponse response = httpClient.execute(httpPost);
if (response.getStatusLine().getStatusCode() == HttpStatus.SC_OK) {
result = EntityUtils.toString(response.getEntity(), "utf-8");
log.info("doPostSuccess({}):{}",url, result);
} else {
log.error("doPostFail({}):{}", url, response.getStatusLine().getStatusCode());
}
} catch (Exception e) {
log.error("doPostError({}):{}", url,ExceptionUtils.getStackTrace(e));
e.printStackTrace();
} finally {
if (null != httpPost) {
// 释放连接
httpPost.releaseConnection();
}
}
return result;
}
2.3 POST-Json
public static String sendJson (String url, JSONObject jsonObject) {
String result = "";
/**
* RequestConfig:
* setSocketTimeout: socket读取数据超时时间
* setConnectTimeout: 与服务器链接超时时间
* setConnectionRequestTimeout: 从连接池获取连接的超时时间
*/
RequestConfig reqConfig = RequestConfig.custom()
.setSocketTimeout(60 * 1000)
.setConnectTimeout(10 * 1000)
.setConnectionRequestTimeout(10 * 1000)
.build();
/**
* HttpClientBuilder:
* setMaxConnTotal: 连接池最大连接数
* setMaxConnPerRoute: 分配给同一个路由最大的并发连接数
* setDefaultConfig(RequestConfig)
*/
HttpClient httpClient = HttpClientBuilder.create()
.setDefaultRequestConfig(reqConfig)
.build();
HttpPost httpPost = new HttpPost(url);
try {
httpPost.addHeader("Content-type", "application/json;charset=utf-8");
httpPost.setHeader("Accept", "application/json");
if (StringUtils.isNotBlank(jsonObject.toString())) {
httpPost.setEntity(new StringEntity(jsonObject.toString(), StandardCharsets.UTF_8));
}
HttpResponse response = httpClient.execute(httpPost);
if (response.getStatusLine().getStatusCode() == HttpStatus.SC_OK) {
HttpEntity entity = response.getEntity();
if (entity != null){
// result = EntityUtils.toString(entity, "utf-8");
result = JSONObject.parseObject(EntityUtils.toString(entity, StandardCharsets.UTF_8)).toJSONString();
}
}else {
log.error("sendJsonFail({}):{}",url, response.getStatusLine().getStatusCode());
}
} catch (Exception e) {
log.error("sendJsonError({}):{}",url, ExceptionUtils.getStackTrace(e));
}finally {
if (null != httpPost) {
// 释放连接
httpPost.releaseConnection();
}
}
return result;
}
2.4 POST-File
public static String sendFilePost(String url, Map<String, ContentBody> reqParam){
CloseableHttpClient httpclient = HttpClients.createDefault();
CloseableHttpResponse response = null;
try {
// 创建http
HttpPost httppost = new HttpPost(url);
/**
* RequestConfig:
* setSocketTimeout: socket读取数据超时时间
* setConnectTimeout: 与服务器链接超时时间
* setConnectionRequestTimeout: 从连接池获取连接的超时时间
*/
RequestConfig reqConfig = RequestConfig.custom()
.setSocketTimeout(60 * 1000)
.setConnectTimeout(10 * 1000)
.setConnectionRequestTimeout(10 * 1000)
.build();
httppost.setConfig(reqConfig);
MultipartEntityBuilder multipartEntityBuilder = MultipartEntityBuilder.create();
multipartEntityBuilder.setMode(HttpMultipartMode.BROWSER_COMPATIBLE);
multipartEntityBuilder.setCharset(StandardCharsets.UTF_8);
for(Map.Entry<String,ContentBody> param : reqParam.entrySet()){
multipartEntityBuilder.addPart(param.getKey(), param.getValue());
}
HttpEntity reqEntity = multipartEntityBuilder.build();
httppost.setEntity(reqEntity);
try {
// 发起post请求
response = httpclient.execute(httppost);
// 获取响应实体
HttpEntity entity = response.getEntity();
if (entity != null) {
// return EntityUtils.toString(entity, StandardCharsets.UTF_8);
// unicode转中文
return JSONObject.parseObject(EntityUtils.toString(entity, StandardCharsets.UTF_8)).toJSONString();
}
} catch (IOException e){
e.printStackTrace();
log.error("请求-{}-响应异常:{}", url, String.valueOf(e));
}
} finally {
// 关闭连接 释放资源
try {
if (response != null){
response.close();
}
httpclient.close();
} catch (IOException e) {
e.printStackTrace();
log.error("请求-{}-内部异常:{}", url, String.valueOf(e));
}
}
return null;
}
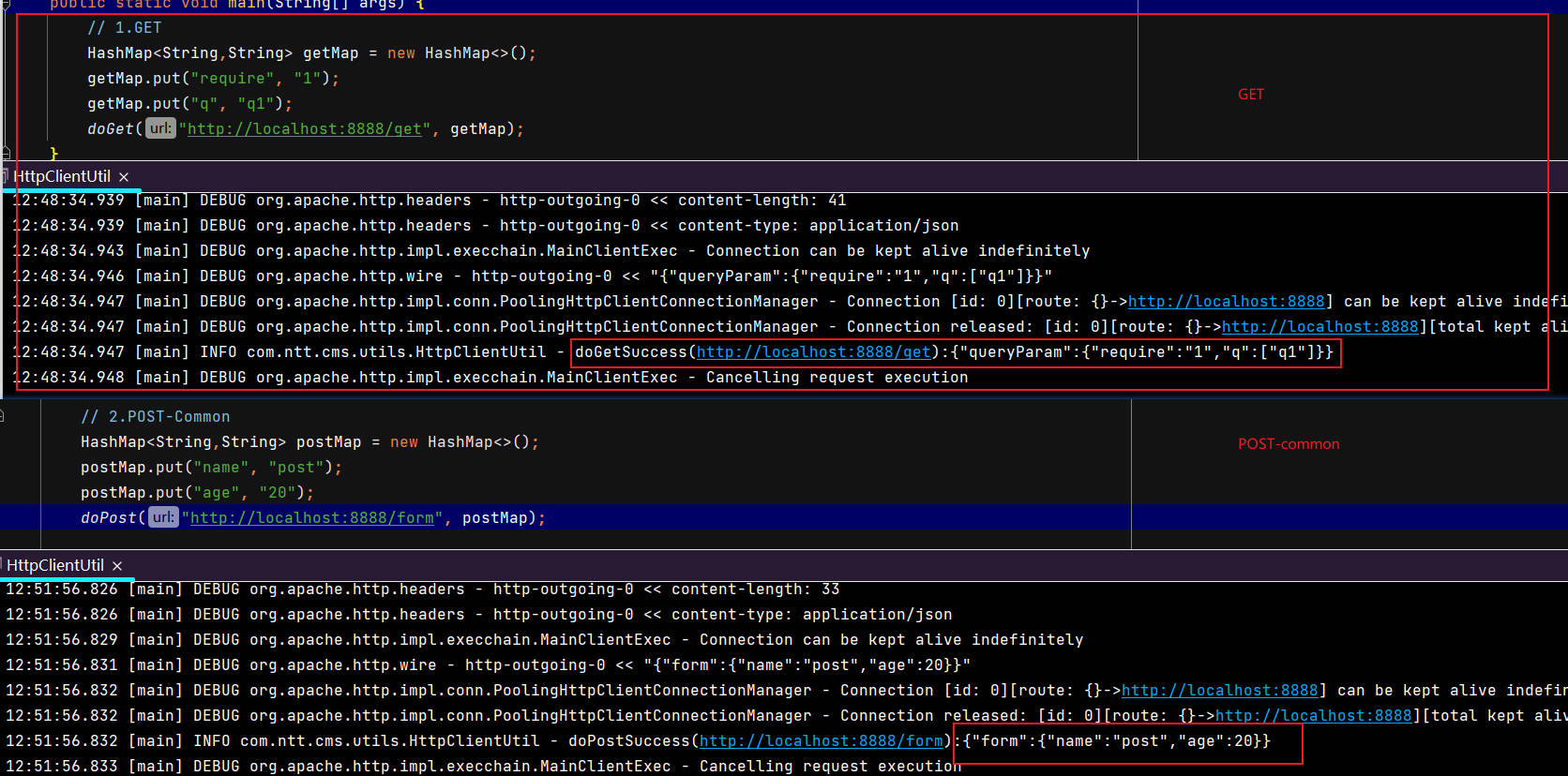
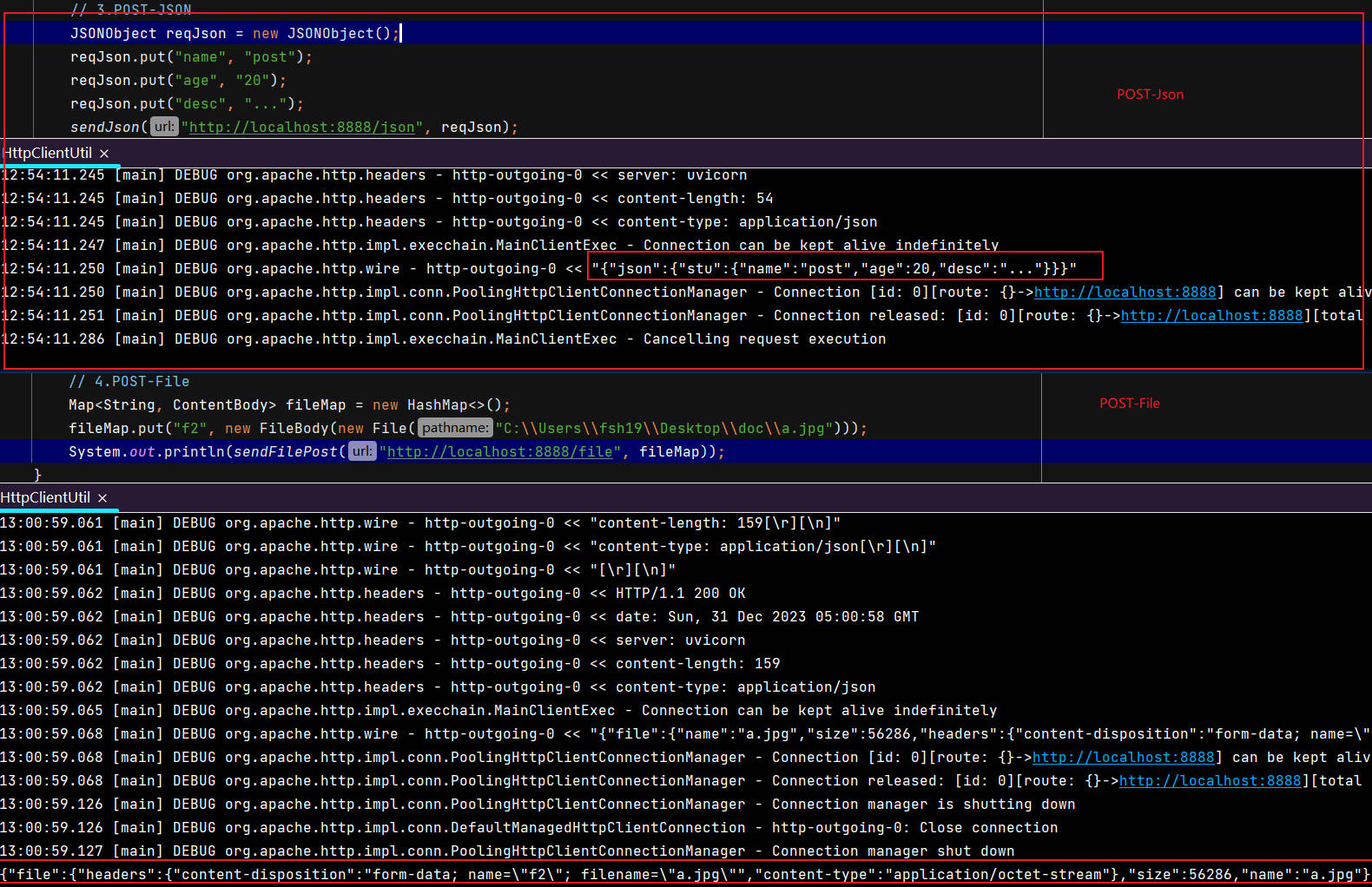