一个简单的QML滚动字幕实现
目录结构
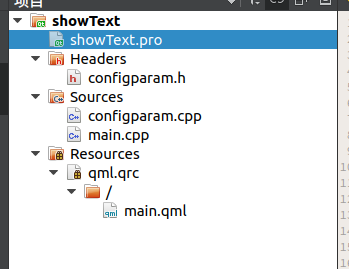
configparam.h
#ifndef CONFIGPARAM_H
#define CONFIGPARAM_H
#include <QObject>
#include <QTypeInfo>
class configParam : public QObject
{
Q_OBJECT
Q_PROPERTY(QString backGroundColor READ getBackGroundColor NOTIFY backGroundColorChanged)
Q_PROPERTY(QString text READ getText NOTIFY textChanged)
Q_PROPERTY(QString textColor READ getTextColor NOTIFY textColorChanged)
Q_PROPERTY(int textSize READ getTextSize NOTIFY textSizeChanged)
Q_PROPERTY(int textWeight READ getTextWeight NOTIFY textWeightChanged)
Q_PROPERTY(int screenWidth READ getScreenWidth NOTIFY screenWidthChanged)
Q_PROPERTY(int screenHeight READ getScreenHeight NOTIFY screenHeightChanged)
Q_PROPERTY(double wordSpacing READ getWordSpacing NOTIFY wordSpacingChanged)
Q_PROPERTY(bool runEnabled READ getRunEnabled NOTIFY runEnabledChanged)
Q_PROPERTY(int duration READ getDuration NOTIFY durationChanged)
signals:
void backGroundColorChanged();
void textChanged();
void textColorChanged();
void textSizeChanged();
void textWeightChanged();
void screenWidthChanged();
void screenHeightChanged();
void wordSpacingChanged();
void runEnabledChanged();
void durationChanged();
public:
configParam(QString filename);
QString getBackGroundColor();
QString getText();
QString getTextColor();
int getTextSize();
int getTextWeight();
int getScreenWidth();
int getScreenHeight();
double getWordSpacing();
bool getRunEnabled();
int getDuration();
private:
QString backGroundColor;
QString text;
QString textColor;
int textSize;
int textWeight;
int screenWidth;
int screenHeight;
double wordSpacing;
bool runEnabled;
int duration;
};
#endif // CONFIGPARAM_H
configparam.cpp
#include "configparam.h"
#include <QSettings>
#include <QDebug>
#include <QTextCodec>
configParam::configParam(QString filename)
{
QSettings settings(filename, QSettings::IniFormat);
settings.setIniCodec(QTextCodec::codecForName("utf-8"));
backGroundColor = settings.value("common/backGroundColor").toString();
text = settings.value("common/text").toString();
textColor = settings.value("common/textColor").toString();
textSize = settings.value("common/textSize").toInt();
textWeight = settings.value("common/textWeight").toInt();
screenWidth = settings.value("common/screenWidth").toInt();
screenHeight = settings.value("common/screenHeight").toInt();
wordSpacing = settings.value("common/wordSpacing").toDouble();
runEnabled = settings.value("common/runEnabled").toBool();
duration = settings.value("common/duration").toInt();
qDebug()<<backGroundColor << text <<textColor << textSize << textWeight <<screenWidth<<screenHeight<<wordSpacing<<runEnabled;
}
QString configParam::getBackGroundColor()
{
return backGroundColor;
}
QString configParam::getText()
{
return text;
}
QString configParam::getTextColor()
{
return textColor;
}
int configParam::getTextSize()
{
return textSize;
}
int configParam::getTextWeight()
{
return textWeight;
}
int configParam::getScreenWidth()
{
return screenWidth;
}
int configParam::getScreenHeight()
{
return screenHeight;
}
double configParam::getWordSpacing()
{
return wordSpacing;
}
bool configParam::getRunEnabled()
{
return runEnabled;
}
int configParam::getDuration()
{
return duration;
}
main.cpp
#include <QGuiApplication>
#include <QQmlApplicationEngine>
#include <QQmlContext>
#include <QFile>
#include <QDebug>
#include "configparam.h"
int main(int argc, char *argv[])
{
QCoreApplication::setAttribute(Qt::AA_EnableHighDpiScaling);
QGuiApplication app(argc, argv);
QString fileName = QCoreApplication::applicationDirPath() + "/config.ini";
if(!QFile::exists(fileName)){
qDebug() << "File not found:" << fileName;
return -1;
}
configParam param(fileName);
QQmlApplicationEngine engine;
const QUrl url(QStringLiteral("qrc:/main.qml"));
QObject::connect(&engine, &QQmlApplicationEngine::objectCreated,
&app, [url](QObject *obj, const QUrl &objUrl) {
if (!obj && url == objUrl)
QCoreApplication::exit(-1);
}, Qt::QueuedConnection);
auto context = engine.rootContext();
context->setContextProperty("configParam",¶m);
engine.load(url);
return app.exec();
}
main.qml
import QtQuick 2.9
import QtQuick.Window 2.3
Window {
id: root
visible: true
width: configParam.screenWidth
height: configParam.screenHeight
// title: qsTr("Hello World")
flags: Qt.FramelessWindowHint
Item{
anchors.fill: parent
Rectangle{
anchors.fill: parent
anchors.centerIn: parent
color: configParam.backGroundColor
Text {
id: txt
anchors.verticalCenter: parent.verticalCenter
anchors.horizontalCenter: parent.horizontalCenter
text: configParam.text
color: configParam.textColor
font.pixelSize: configParam.textSize
font.weight: configParam.textWeight
font.wordSpacing: configParam.wordSpacing
font.bold: true
// font.family: "Times New Roman"
XAnimator {
id:animation
target: txt
running: configParam.runEnabled
loops: Animation.Infinite
from: root.width
to: -txt.width
duration: configParam.duration
}
}
}
}
}
Qt .pro文件
QT += quick
CONFIG += c++11
# The following define makes your compiler emit warnings if you use
# any Qt feature that has been marked deprecated (the exact warnings
# depend on your compiler). Refer to the documentation for the
# deprecated API to know how to port your code away from it.
DEFINES += QT_DEPRECATED_WARNINGS
# You can also make your code fail to compile if it uses deprecated APIs.
# In order to do so, uncomment the following line.
# You can also select to disable deprecated APIs only up to a certain version of Qt.
#DEFINES += QT_DISABLE_DEPRECATED_BEFORE=0x060000 # disables all the APIs deprecated before Qt 6.0.0
SOURCES += \
main.cpp \
configparam.cpp
RESOURCES += qml.qrc
# Additional import path used to resolve QML modules in Qt Creator's code model
QML_IMPORT_PATH =
# Additional import path used to resolve QML modules just for Qt Quick Designer
QML_DESIGNER_IMPORT_PATH =
# Default rules for deployment.
qnx: target.path = /tmp/$${TARGET}/bin
else: unix:!android: target.path = /opt/$${TARGET}/bin
!isEmpty(target.path): INSTALLS += target
HEADERS += \
configparam.h
config.ini配置文件
[common]
#设置屏幕分辨率宽/高
screenWidth=1920
screenHeight=600
#设置背景色,纯色包括,black,white,green,blue等
backGroundColor="black"
#设置显示的文字内容
text="欢迎XX领导莅临参观指导"
#设置字体大小与粗细
textSize=150
textWeight=100
#设置字体颜色,纯色包括,black,white,green,blue等
textColor="red"
#设置字间距
wordSpacing=0
#设置是否滚动显示
runEnabled=true
#设置滚动时长(速度),单位ms
duration=10000