练习用的
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script src="https://cdn.jsdelivr.net/npm/vue@2/dist/vue.js"></script>
<!-- 百度jqueryCDN-->
<script src="https://apps.bdimg.com/libs/jquery/2.1.4/jquery.min.js"></script>
<!-- 最新版本的 Bootstrap 核心 CSS 文件 -->
<link rel="stylesheet" href="https://cdn.bootcdn.net/ajax/libs/twitter-bootstrap/3.4.1/css/bootstrap.min.css"
integrity="sha384-HSMxcRTRxnN+Bdg0JdbxYKrThecOKuH5zCYotlSAcp1+c8xmyTe9GYg1l9a69psu" crossorigin="anonymous">
<!-- 可选的 Bootstrap 主题文件(一般不用引入) -->
<link rel="stylesheet" href="https://cdn.bootcdn.net/ajax/libs/twitter-bootstrap/3.4.1/css/bootstrap-theme.min.css"
integrity="sha384-6pzBo3FDv/PJ8r2KRkGHifhEocL+1X2rVCTTkUfGk7/0pbek5mMa1upzvWbrUbOZ" crossorigin="anonymous">
<!-- 最新的 Bootstrap 核心 JavaScript 文件 -->
<script src="https://cdn.bootcdn.net/ajax/libs/twitter-bootstrap/3.4.1/js/bootstrap.min.js"
integrity="sha384-aJ21OjlMXNL5UyIl/XNwTMqvzeRMZH2w8c5cRVpzpU8Y5bApTppSuUkhZXN0VxHd"
crossorigin="anonymous"></script>
<style>
.panel.panel-default {
margin: 6px; /* 添加外边距,可以根据需要调整像素值 */
}
</style>
</head>
<body>
<div id="app">
<h3>表单区域</h3>
<div>
<div>
<label for="id1">姓名</label>
<input type="text" name="" id="id1" v-model="user">
</div>
<div>
<label for="id2">年龄</label>
<input type="text" name="" id="id2" v-model="age">
</div>
<input type="button" :value="title" v-on:click="addUser">
</div>
<h3>数据列表</h3>
<div class="panel panel-default">
<table class="table">
<thead class="panel-heading">
<tr>
<td>姓名</td>
<td>年龄</td>
<td>删除</td>
</tr>
</thead>
<tbody class="panel-body">
<tr v-for="(item, idx) in dataList">
<td>{{item.name}}</td>
<td>{{item.age}}</td>
<td>
<input type="button" value="删除" v-on:click="del(idx)">
<input type="button" value="编辑" v-on:click="edit" :data-idx="idx">
</td>
</tr>
<!-- <tr>-->
<!-- <td>麻子</td>-->
<!-- <td>50</td>-->
<!-- </tr>-->
</tbody>
</table>
</div>
</div>
<script>
var app = new Vue({
el: '#app',
data: {
editIndex: undefined,
title: "新建",
user: "",
age: "",
dataList: [
{"name": "张三", "age": 20},
{"name": "麻子", "age": 30},
]
},
methods: {
addUser: function () {
if (this.editIndex) {
// 新增
this.dataList[this.editIndex].name = this.user;
this.dataList[this.editIndex].age = this.age;
alert("修改成功!")
this.title = "新建"; // 按钮恢复原装
this.editIndex = undefined;
} else {
if ((!this.user.trim()) || (!this.age.trim())) {
alert("请输入正确的格式!")
} else {
// 新增
this.dataList.push({"name": this.user, "age": this.age})
// this.user='';
// this.age='';
}
}
this.user = '';
this.age = '';
},
del: function (idx) {
// 数组中删除特定的对象,可以使用splice方法或filter方法 也可以用event.target.dataset.xxx
this.dataList.splice(idx, 1)
},
edit: function (event) {
let idx = event.target.dataset.idx
// let name = this.dataList[idx].name
// let age = this.dataList[idx].age
let {name, age} = this.dataList[idx] // 类似于python的解包
this.user = name;
this.age = age;
this.title = "编辑";
this.editIndex = idx;
}
}
})
</script>
</body>
</html>
图
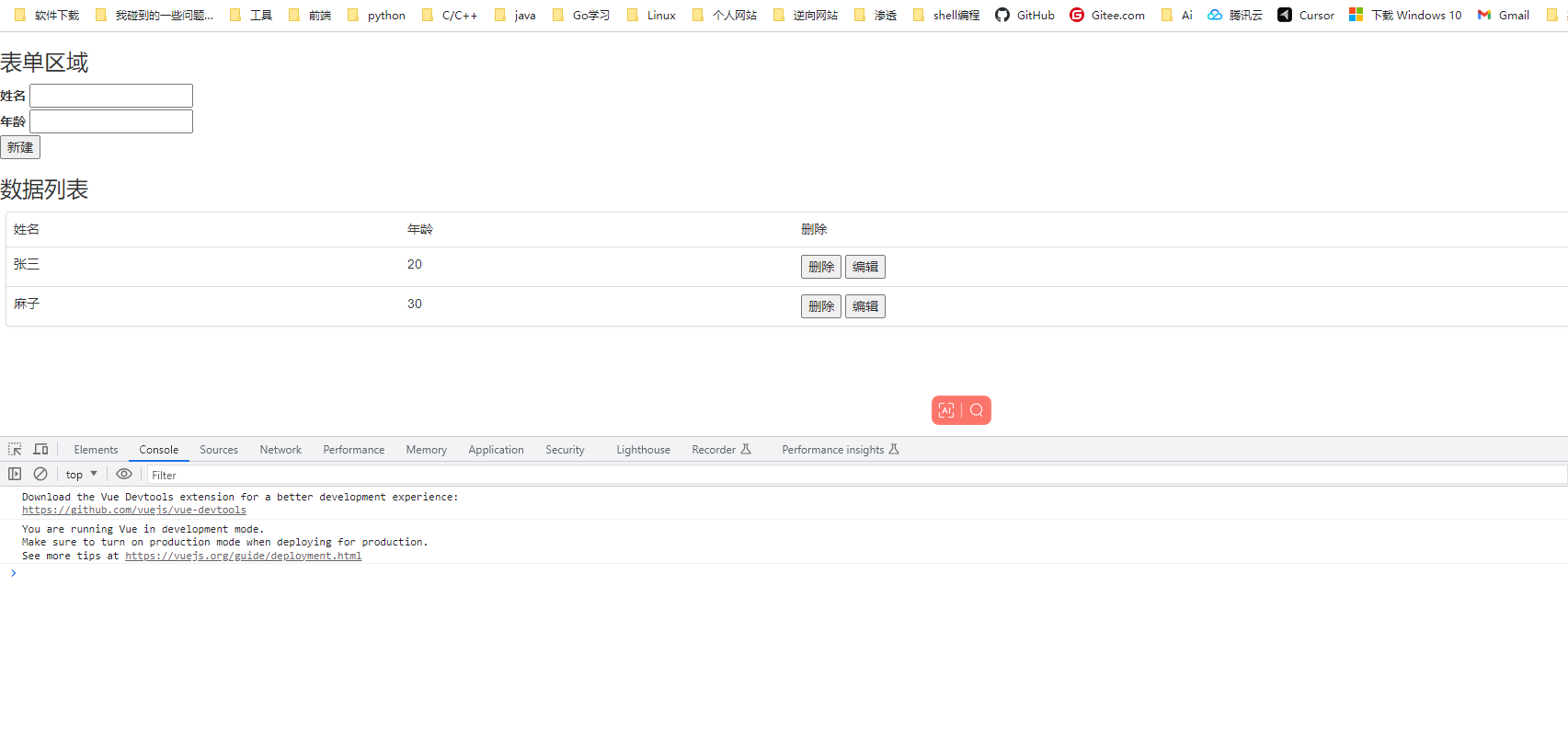