L2-033 简单计算器
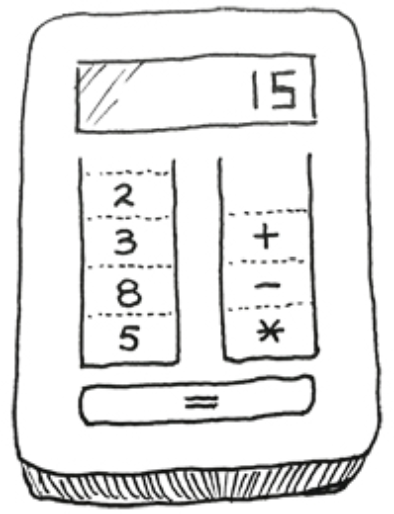
本题要求你为初学数据结构的小伙伴设计一款简单的利用堆栈执行的计算器。如上图所示,计算器由两个堆栈组成,一个堆栈 \(S_{1}\); 存放数字,另一个堆栈 \(S_{2}\) 存放运算符。计算器的最下方有一个等号键,每次按下这个键,计算器就执行以下操作:
从 \(S_{1}\) 中弹出两个数字,顺序为 \(n_{1}\) 和 \(n_{2}\);
从 \(S_{2}\) 中弹出一个运算符 \(op\) ;
执行计算 \(n_{2}\) \(op\) \(n_{1}\);
将得到的结果压回 \(S_{1}\) 。
直到两个堆栈都为空时,计算结束,最后的结果将显示在屏幕上。
输入格式:
输入首先在第一行给出正整数 \(N\)( \(1<N≤10^{3}\) ),为 \(S_{1}\) 中数字的个数。
第二行给出 \(N\) 个绝对值不超过 100 的整数;第三行给出 \(N−1\) 个运算符 —— 这里仅考虑 \(+、-、*、/\) 这四种运算。一行中的数字和符号都以空格分隔。
输出格式:
将输入的数字和运算符按给定顺序分别压入堆栈 \(S_{1}\) 和 \(S_{2}\) ,将执行计算的最后结果输出。注意所有的计算都只取结果的整数部分。题目保证计算的中间和最后结果的绝对值都不超过 \(10^{9}\) 。
如果执行除法时出现分母为零的非法操作,则在一行中输出:\(ERROR:\) \(X/0\),其中 \(X\) 是当时的分子。然后结束程序。
输入样例 1:
5
40 5 8 3 2
/ * - +
输出样例 1:
2
输入样例 2:
5
2 5 8 4 4
* / - +
输出样例 2:
ERROR: 5/0
解题思路
先将所有数字先放入栈\(num\)中,把所有字符放入栈\(ops\)中,然后进行模拟,直到最后栈\(num\)的大小为1,此时栈中的数字就是最后的答案。但是,可能会存在除以0的情况,我们只需要把第一次出现这个错误的情况记录下来,若出现错误,输出 \(ERROR:\) \(X/0\),其中 \(X\) 是当时的分子。
/* 一切都是命运石之门的选择 El Psy Kongroo */
#include<iostream>
#include<cstdio>
#include<cstring>
#include<string>
#include<algorithm>
#include<vector>
#include<queue>
#include<deque>
#include<stack>
#include<map>
#include<set>
#include<bitset>
#include<cmath>
#include<functional>
using namespace std;
typedef long long ll;
typedef unsigned long long ull;
typedef pair<int, int> pii;
typedef pair<int, pii> piii;
typedef pair<double, double> pdd;
typedef pair<string, int> psi;
typedef __int128 int128;
#define PI acos(-1.0)
#define x first
#define y second
//int dx[4] = {1, -1, 0, 0};
//int dy[4] = {0, 0, 1, -1};
const int inf = 0x3f3f3f3f, mod = 1e9 + 7;
int n;
stack<int> num;
stack<char> ops;
bool flag = true;
int error; //标记错误
int get_res(int n1, int n2, int op){
int res = 0;
if(op == '+') res = n1 + n2;
else if(op == '-') res = n2 - n1;
else if(op == '*') res = n2 * n1;
else if(op == '/'){
if(n1 == 0){
if(flag){
flag = false;
error = n2; //只记录第一次错误
res = inf;
}
}else res = n2 / n1;
}
return res;
}
int main(){
ios::sync_with_stdio(false);
cin.tie(0), cout.tie(0);
cin >> n;
for(int i = 0; i < n; i ++ ){
int x; cin >> x;
num.push(x);
}
for(int i = 0; i < n - 1; i ++ ){
char ch; cin >> ch;
ops.push(ch);
}
while((int)num.size() > 1){
int n1 = num.top();
num.pop();
int n2 = num.top();
num.pop();
char op = ops.top();
ops.pop();
int res = get_res(n1, n2, op);
num.push(res);
}
if(flag) cout << num.top() << endl;
else cout << "ERROR: " << error << "/0" << endl;
return 0;
}