外观模式(Facade Pattern)
一、定义
外观模式(Facade Pattern)隐藏系统的复杂性,并向客户端提供了一个客户端可以访问系统的接口。这种类型的设计模式属于结构型模式,它向现有的系统添加一个接口,来隐藏系统的复杂性。
这种模式涉及到一个单一的类,该类提供了客户端请求的简化方法和对现有系统类方法的委托调用。
二、优缺点
优点: 1、减少系统相互依赖。 2、提高灵活性。 3、提高了安全性。
缺点: 不符合开闭原则,如果要改东西很麻烦,继承重写都不合适。
三、具体实现
3.1 原型图
- Facade 外观角色
- Subsystem classes 子系统角色
- client 客户端角色
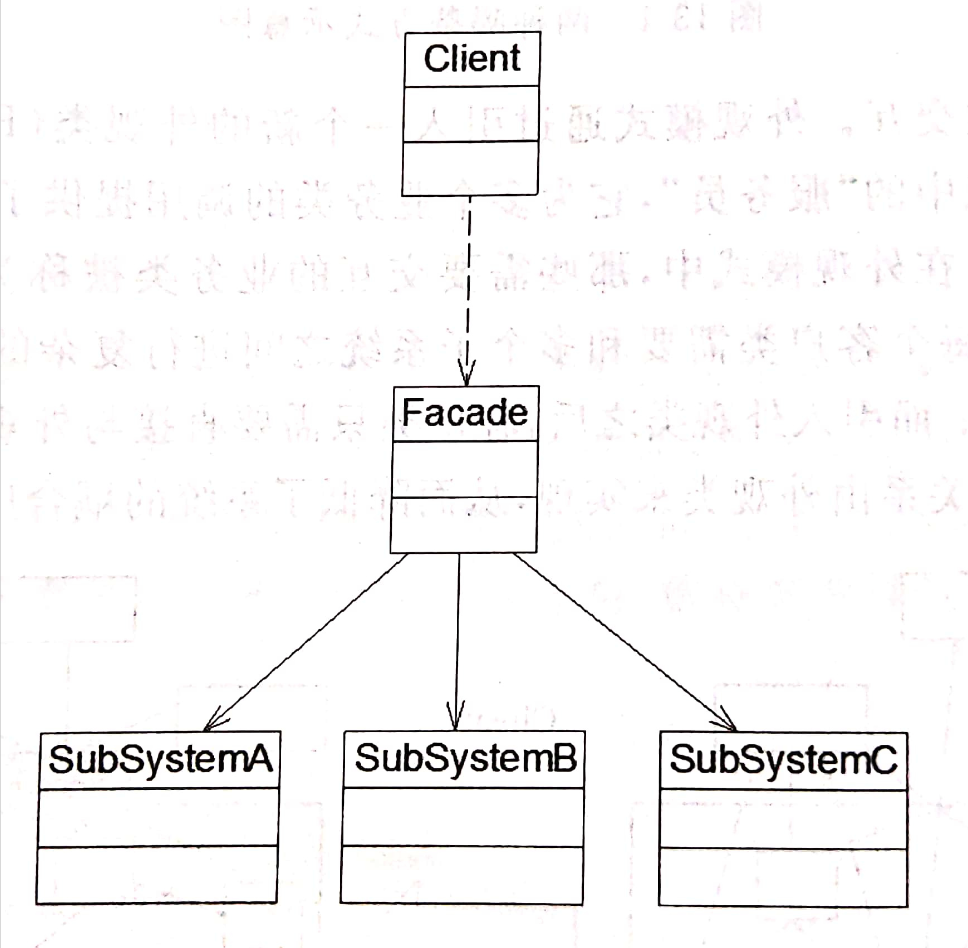
3.2 实现
1、创建一个接口。
/**
* @author zhongtao
* @date 2023/5/23 21:23
*/
public interface Shape {
void draw();
}
2、创建实现接口的实体类。
/**
* @author zhongtao
* @date 2023/5/23 21:24
*/
public class Circle implements Shape {
@Override
public void draw() {
System.out.println("Circle::draw()");
}
}
/**
* @author zhongtao
* @date 2023/5/23 21:23
*/
public class Rectangle implements Shape {
@Override
public void draw() {
System.out.println("Rectangle::draw()");
}
}
/**
* @author zhongtao
* @date 2023/5/23 21:23
*/
public class Square implements Shape {
@Override
public void draw() {
System.out.println("Square::draw()");
}
}
3、创建一个外观类。
/**
* @author zhongtao
* @date 2023/5/23 21:24
*/
public class ShapeMaker {
private Shape circle;
private Shape rectangle;
private Shape square;
public ShapeMaker() {
circle = new Circle();
rectangle = new Rectangle();
square = new Square();
}
public void drawCircle() {
circle.draw();
}
public void drawRectangle() {
rectangle.draw();
}
public void drawSquare() {
square.draw();
}
}
4、使用该外观类画出各种类型的形状。
/**
* @author zhongtao
* @date 2023/5/23 21:24
*/
public class FacadePatternDemo {
public static void main(String[] args) {
ShapeMaker shapeMaker = new ShapeMaker();
shapeMaker.drawCircle();
shapeMaker.drawRectangle();
shapeMaker.drawSquare();
}
}
5、执行程序,输出结果:
Circle::draw()
Rectangle::draw()
Square::draw()