内容比较简单,和其他院的上机比起来说是这样的
实现二叉树的基本操作,二叉树使用链式结构建立,基本操作基本用递归实现
1. 问题描述
二叉树的基本操作;
(1)创建二叉树,需注意此处是按照先序法输入
(2)通过先序遍历、中序遍历、后序遍历分别输出二叉树
(3)求取二叉树的结点总数、树的深度
2.数据结构设计
二叉树,用链式结构
3.算法设计
按照要求写出对应函数实现功能即可,包括先序输入,遍历和求结点数、树深度等.一般都是递归实现
4.运行、测试与分析
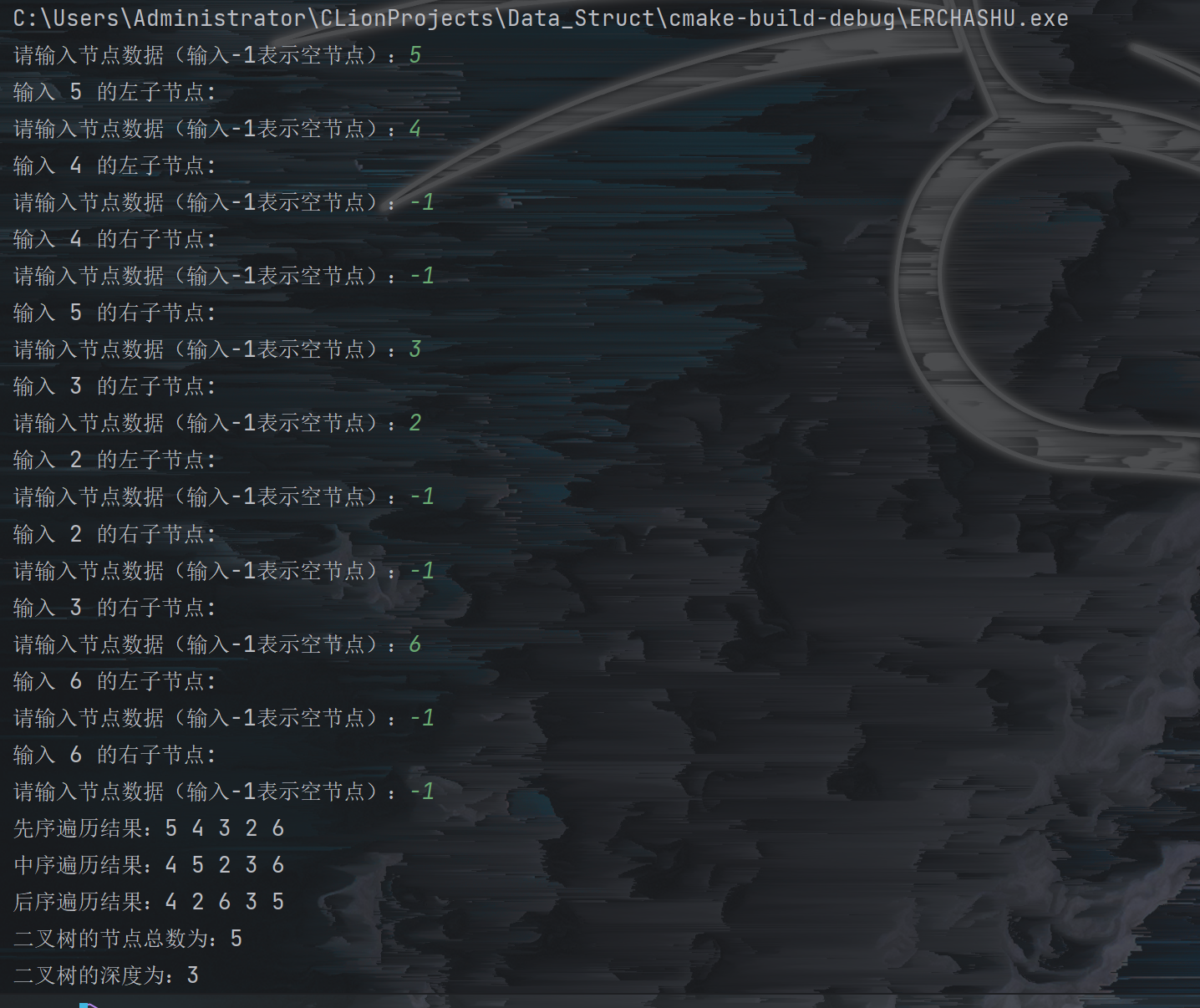
5.附录:源代码
1 #include <stdio.h> 2 #include <stdlib.h> 3 4 // 二叉树节点定义 5 typedef struct Node { 6 int data; 7 struct Node* left; 8 struct Node* right; 9 } Node; 10 11 // 创建二叉树 12 Node* createBinaryTree() { 13 int data; 14 printf("请输入节点数据(输入-1表示空节点):"); 15 scanf("%d", &data); 16 17 if (data == -1) { 18 return NULL; 19 } 20 21 Node* newNode = (Node*)malloc(sizeof(Node)); 22 newNode->data = data; 23 24 printf("输入 %d 的左子节点:\n", data); 25 newNode->left = createBinaryTree(); 26 27 printf("输入 %d 的右子节点:\n", data); 28 newNode->right = createBinaryTree(); 29 30 return newNode; 31 } 32 33 // 先序遍历二叉树 34 void preOrderTraversal(Node* root) { 35 if (root != NULL) { 36 printf("%d ", root->data); 37 preOrderTraversal(root->left); 38 preOrderTraversal(root->right); 39 } 40 } 41 42 // 中序遍历二叉树 43 void inOrderTraversal(Node* root) { 44 if (root != NULL) { 45 inOrderTraversal(root->left); 46 printf("%d ", root->data); 47 inOrderTraversal(root->right); 48 } 49 } 50 51 // 后序遍历二叉树 52 void postOrderTraversal(Node* root) { 53 if (root != NULL) { 54 postOrderTraversal(root->left); 55 postOrderTraversal(root->right); 56 printf("%d ", root->data); 57 } 58 } 59 60 // 计算二叉树节点总数 61 int countNodes(Node* root) { 62 if (root == NULL) { 63 return 0; 64 } 65 66 return 1 + countNodes(root->left) + countNodes(root->right); 67 } 68 /* 69 这是一个用于计算二叉树节点数量的递归函数。函数接收一个指向二叉树根节点的指针 root,并返回二叉树中节点的总数。 70 首先,函数会检查传入的根节点是否为空(NULL)。如果是空节点,则表示当前子树为空,直接返回0作为节点数。 71 如果根节点不为空,函数将会进行递归调用。它通过分别对左子树和右子树调用 countNodes 函数来计算它们各自的节点数,并将结果相加。然后再加上当前根节点本身,最终得到整个二叉树的节点数。 72 可以通过调用该函数并传入二叉树的根节点来获取二叉树中的节点总数。 73 */ 74 75 // 计算二叉树的深度 76 int calculateDepth(Node* root) { 77 if (root == NULL) { 78 return 0; 79 } 80 81 int leftDepth = calculateDepth(root->left); 82 int rightDepth = calculateDepth(root->right); 83 84 return (leftDepth > rightDepth ? leftDepth : rightDepth) + 1; 85 } 86 /* 87 这是一个计算二叉树深度的递归函数。它接受一个指向根节点的指针,然后通过递归的方式计算左子树和右子树的深度,并返回较大值加1作为整棵树的深度。 88 首先,函数会检查根节点是否为空,如果为空则返回0表示空树的深度为0。 89 然后,它分别调用 calculateDepth 函数来计算左子树和右子树的深度,并将结果保存在 leftDepth 和 rightDepth 变量中。 90 最后,函数会比较左子树和右子树的深度大小,并将较大值加1作为整棵树的深度返回。 91 @return 92 */ 93 int main() { 94 Node* root = createBinaryTree(); 95 96 printf("先序遍历结果:"); 97 preOrderTraversal(root); 98 99 printf("\n中序遍历结果:"); 100 inOrderTraversal(root); 101 102 printf("\n后序遍历结果:"); 103 postOrderTraversal(root); 104 105 int nodeCount = countNodes(root); 106 printf("\n二叉树的节点总数为:%d\n", nodeCount); 107 108 int depth = calculateDepth(root); 109 printf("二叉树的深度为:%d\n", depth); 110 111 return 0; 112 }