在一个排序的链表中,存在重复的结点,请删除该链表中重复的结点,重复的结点不保留,返回链表头指针。 例如,链表 1->2->3->3->4->4->5 处理后为 1->2->5
数据范围:链表长度满足 0≤n≤1000,链表中的值满足 1≤val≤1000
进阶:空间复杂度 O(n),时间复杂度 O(n)
例如输入{1,2,3,3,4,4,5}时,对应的输出为{1,2,5},对应的输入输出链表如下图所示:
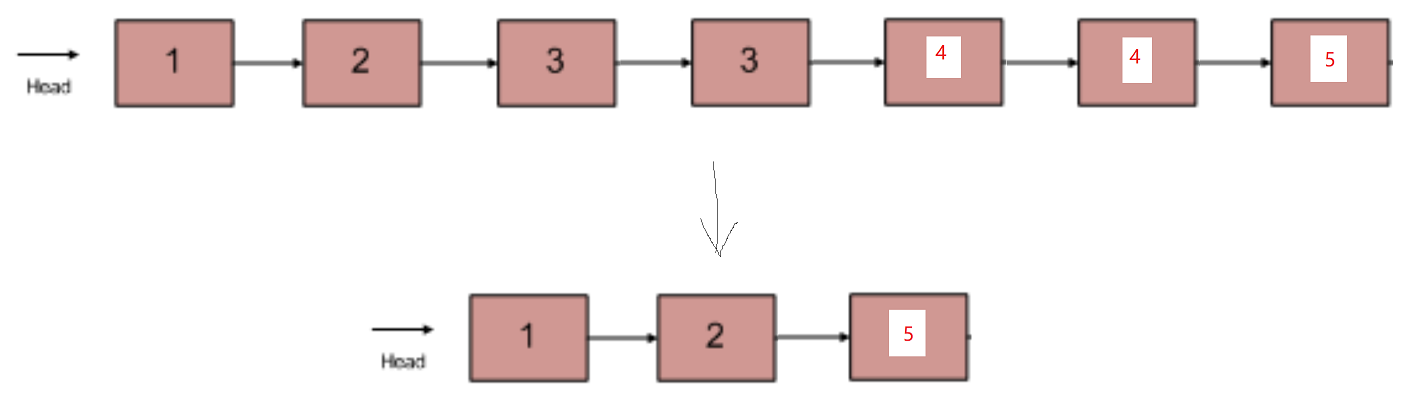
示例1
输入:{1,2,3,3,4,4,5}输出:{1,2,5}
示例2
输入:{1,1,1,8}输出:{8}
public class Solution { public ListNode deleteDuplication(ListNode pHead) { //空链表 if(pHead == null) return null; ListNode res = new ListNode(0); //在链表前加一个表头 res.next = pHead; ListNode cur = res; while(cur.next != null && cur.next.next != null){ //遇到相邻两个节点值相同 if(cur.next.val == cur.next.next.val){ int temp = cur.next.val; //将所有相同的都跳过 while (cur.next != null && cur.next.val == temp) { cur.next = cur.next.next; } } else { cur = cur.next; } } //返回时去掉表头 return res.next; } }