具体代码实现
#include<iostream>
using namespace std;
class Man {
public:
virtual void show() = 0;
};
class Woman {
public:
virtual void show() = 0;
};
class YellowMan : public Man {
public:
virtual void show() {
cout << "黄皮肤的男性" << endl;
}
};
class BlackMan : public Man {
public:
virtual void show() {
cout << "黑皮肤的男性" << endl;
}
};
class WhiteMan : public Man {
public:
virtual void show() {
cout << "白皮肤的男性" << endl;
}
};
class YellowWoman : public Woman {
public:
virtual void show() {
cout << "黄皮肤的女性" << endl;
}
};
class BlackWoman : public Woman {
public:
virtual void show() {
cout << "黑皮肤的女性" << endl;
}
};
class WhiteWoman : public Woman {
public:
virtual void show() {
cout << "白皮肤的女性" << endl;
}
};
class AbstractFactory {
public:
virtual Man* produceMan() = 0;
virtual Woman* produceWoman() = 0;
};
class YellowFactory : public AbstractFactory {
public:
virtual Man* produceMan() {
return new YellowMan();
}
virtual Woman* produceWoman() {
return new YellowWoman();
}
};
class BlackFactory : public AbstractFactory {
public:
virtual Man* produceMan() {
return new BlackMan();
}
virtual Woman* produceWoman() {
return new BlackWoman();
}
};
class WhiteFactory : public AbstractFactory {
public:
virtual Man* produceMan() {
return new WhiteMan();
}
virtual Woman* produceWoman() {
return new WhiteWoman();
}
};
int main() {
cout << "请选择:1、白皮肤;2、黑皮肤;3、黄皮肤" << endl;
int i;
cin >> i;
if (i == 1) {
AbstractFactory* factory = NULL;
factory = new YellowFactory;
Man* man = factory->produceMan();
man->show();
delete man;
man = NULL;
Woman* woman = factory->produceWoman();
woman->show();
delete woman;
woman = NULL;
delete factory;
factory = NULL;
}
else if (i == 2) {
AbstractFactory* factory = NULL;
factory = new BlackFactory;
Man *man = factory->produceMan();
man->show();
delete man;
man = NULL;
Woman *woman = factory->produceWoman();
woman->show();
delete woman;
woman = NULL;
delete factory;
factory = NULL;
}
else if (i == 3) {
AbstractFactory* factory = NULL;
factory = new WhiteFactory;
Man *man = factory->produceMan();
man->show();
delete man;
man = NULL;
Woman *woman = factory->produceWoman();
woman->show();
delete woman;
woman = NULL;
delete factory;
factory = NULL;
}
else {
cout << "请输入正确的格式~" << endl;
}
return 0;
}
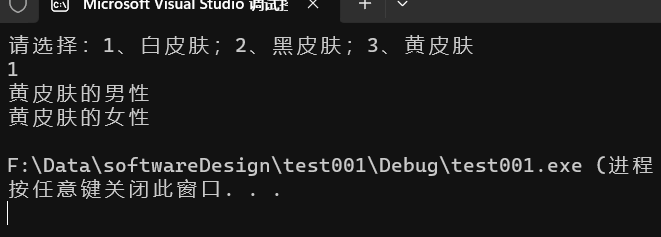
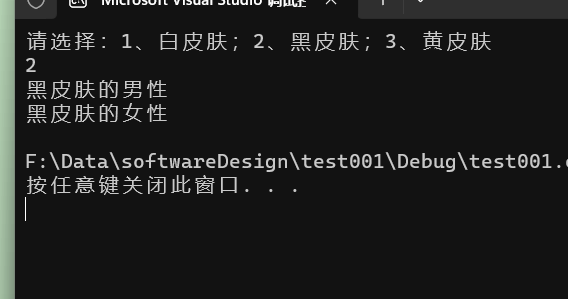
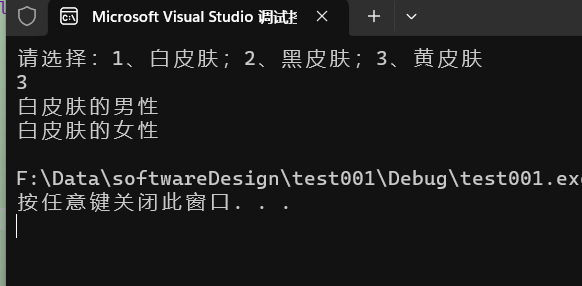
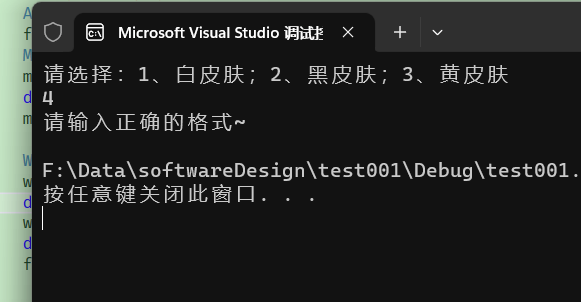