1、效果图
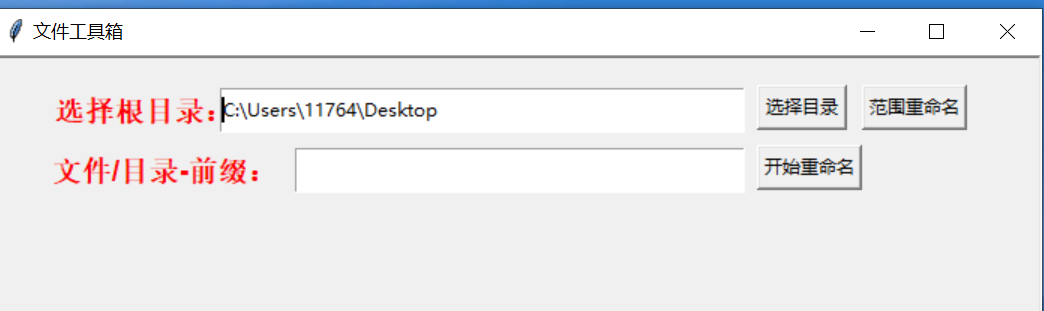
2、代码
import os
from tkinter import *
from tkinter import filedialog, ttk
def init():
root = Tk()
root.title("文件工具箱")
root.geometry("700x400")
frame = Frame(root, relief=SUNKEN, borderwidth=2, width=450, height=250)
frame.pack(side=TOP, fill=BOTH, expand=1)
titleLabel = Label(frame, text="选择根目录:", fg="red", font=("Arial Bold", 14))
titleLabel.place(x=30, y=20, width=140, height=30)
dirPathVar = StringVar()
dirPathVar.set(os.path.abspath('.'))
dirPathEntryBox = Entry(frame, width=70, textvariable=dirPathVar)
dirPathEntryBox.focus() # 自动聚焦
# 设置放置的位置
dirPathEntryBox.place(x=150, y=20, width=350, height=30)
def askDir():
dirName = filedialog.askdirectory()
dirPathVar.set(dirName)
pathSelectedBtn = Button(root, text='选择目录', relief=RAISED, command=askDir)
pathSelectedBtn.place(x=510, y=20, width=60, height=30)
filePrefixLabel = Label(frame, text="文件/目录-前缀:", fg="red", font=("Arial Bold", 14))
filePrefixLabel.place(x=34, y=60, width=160, height=30)
prefixVar = StringVar()
prefixVarEntryBox = Entry(frame, width=70, textvariable=prefixVar)
prefixVarEntryBox.place(x=200, y=60, width=300, height=30)
def savePrefix():
fileNames = []
fileNameTuples = os.walk(dirPathVar.get())
for i, j, fileNameArr in fileNameTuples:
for fileName in fileNameArr:
fileNames.append(fileName)
for idx in range(len(fileNames)):
os.rename(dirPathVar.get() + "/" + fileNames[idx],
dirPathVar.get() + "/" + prefixVar.get() + "-" + fileNames[idx])
prefixBtn = Button(root, text='开始重命名', relief=RAISED, command=savePrefix)
prefixBtn.place(x=510, y=60, width=70, height=30)
def rangeNumRename():
fileNames = []
fileNameTuples = os.walk(dirPathVar.get())
for i, j, fileNameArr in fileNameTuples:
for fileName in fileNameArr:
fileNames.append(fileName)
for idx in range(len(fileNames)):
os.rename(dirPathVar.get() + "/" + fileNames[idx],
dirPathVar.get() + "/" + str(idx + 1) + "-" + fileNames[idx])
startRangeNumBtn = Button(root, text='范围重命名', relief=RAISED, command=rangeNumRename)
startRangeNumBtn.place(x=580, y=20, width=70, height=30)
root.mainloop()
def main():
init()
if __name__ == '__main__':
main()