找链表的中间节点
- 思路
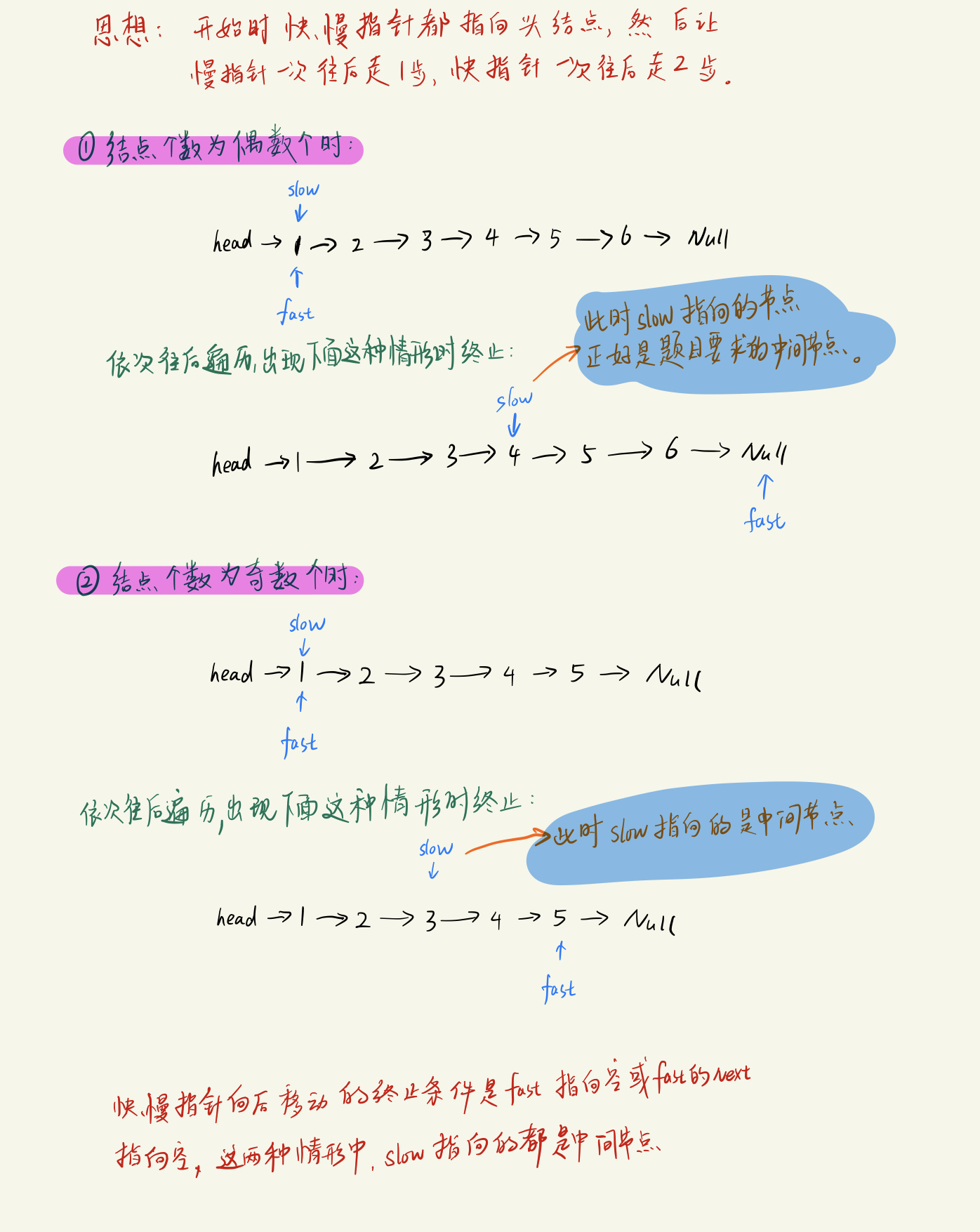
- 心得
- 当不知道while的终止条件时,可以先写while(true),然后在循环体中写终止条件,这样写的好处是可以暂时不考虑终止条件,使思路更清晰;坏处是这样有时候会使循环体的内容很混乱
- 要注意分类!本题中把情况分为节点个数是奇数和偶数去分析,最终找到统一的循环终止条件,就能使代码从第一版简化成第二版
第一版
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode() : val(0), next(nullptr) {}
* ListNode(int x) : val(x), next(nullptr) {}
* ListNode(int x, ListNode *next) : val(x), next(next) {}
* };
*/
class Solution {
public:
ListNode* middleNode(ListNode* head) {
ListNode*slow=head,*fast=head;
while(fast!=NULL){
if(fast->next==NULL)//如果fast已经走到最后的节点了,此时slow应该走到了中间节点处
return slow;
else//如果fast没有走到最后,fast往后走两步
fast=fast->next->next;
if(fast==NULL)
return slow->next;
slow=slow->next;//slow往后走一步
}
return NULL;
}
};
第二版
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode() : val(0), next(nullptr) {}
* ListNode(int x) : val(x), next(nullptr) {}
* ListNode(int x, ListNode *next) : val(x), next(next) {}
* };
*/
class Solution {
public:
ListNode* middleNode(ListNode* head) {
ListNode*slow=head,*fast=head;
while(fast && fast->next){
slow=slow->next;
fast=fast->next->next;
}
return slow;
}
};