1.题目

2.算法思路
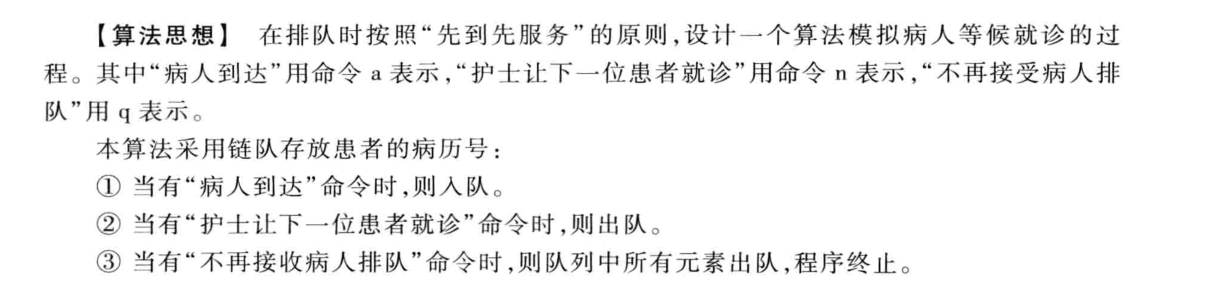
3.代码
//
// Created by trmbh on 2023-09-13.
//
//
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#define TRUE 1
#define FALSE 0
#define MAXSIZE 50
#define QueueElementType int
/* 顺序队列 */
typedef struct {
QueueElementType element[MAXSIZE];
int front;
int rear;
} SeqQueue;
void InitQueue(SeqQueue *Q) {
Q->front = Q->rear = 0;
}
int EnterQueue(SeqQueue *Q, QueueElementType x) {
/* 队列满(如果均用Q->front == Q->rear,那么无法判断队满还是队空,所以牺牲一个存储空间) */
if ((Q->rear + 1) % MAXSIZE == Q->front) return FALSE;
Q->element[Q->rear] = x;
Q->rear = (Q->rear + 1) % MAXSIZE;
return TRUE;
}
int DeleteQueue(SeqQueue *Q, QueueElementType *x) {
/* 队列空 */
if (Q->front == Q->rear) return FALSE;
*x = Q->element[Q->front];
Q->front = (Q->front + 1) % MAXSIZE;
return TRUE;
}
int DestroyQueue(SeqQueue *Q) {
Q->front = Q->rear = 0;
return TRUE;
}
QueueElementType GetHead(SeqQueue *Q) {
return Q->element[Q->front];
}
int IsEmpty(SeqQueue *Q) {
if (Q->front == Q->rear) return TRUE;
else return FALSE;
}
void SeeDoctor(SeqQueue *Q){
int flag = 1;
int n;
char ch;
while (flag){
printf("请输入命令:");
ch = _getch();
switch(ch){
case 'a':
printf("\n请输入病历号:");
scanf("%d", &n);
EnterQueue(Q, n);
break;
case 'n':
if (!IsEmpty(Q)) {
DeleteQueue(Q, &n);
printf("\n病人%d已经就诊\n", n);
} else printf("\n无病人等候\n");
break;
case 'q':
printf("\n今日停止就诊,请等待明日再来\n");
DestroyQueue(Q);
flag = 0;
break;
case 's':
if (!IsEmpty(Q)) {
printf("\n当前等候队列:");
int i = Q->front;
while (i != Q->rear) {
printf(" %d", Q->element[i]);
i = (i + 1) % MAXSIZE;
}
printf("\n");
} else {
printf("\n当前无病人等候.\n");
}
break;
default:
printf("\n非法命令!\n");
}
}
}
int main() {
SeqQueue Q;
InitQueue(&Q);
SeeDoctor(&Q);
DestroyQueue(&Q);
return 0;
}